Unlock the Potential of API Versioning in Django for Enhanced Performance
In the rapidly evolving landscape of web development, maintaining and updating APIs is a critical task for ensuring seamless service and user experience. Particularly in frameworks like Django, API versioning plays a pivotal role. This article delves into the best practices and strategies for managing changes in API endpoints using Django. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. Understanding the Need for API Versioning
API versioning is essential for maintaining backward compatibility while introducing new features or making necessary changes. Without proper versioning, updates can disrupt the user experience and break existing client applications.
2. Why Django for API Development?
Django, a high-level Python web framework, is a popular choice for building robust APIs due to its scalability and versatility. It simplifies the development process by providing a clean and pragmatic design.
Example Case:
Imagine a scenario where an e-commerce platform updates its API for better inventory management. Without versioning, the mobile app relying on the old API might fail to display products correctly.
3. Strategies for API Versioning in Django
3.1. URL Path Versioning
This involves including the version number in the URL path.
Example:
– Version 1: `api/v1/products`
– Version 2: `api/v2/products`
This method is straightforward and easily readable. However, it can lead to URL proliferation with each new version.
3.2. Query Parameter Versioning
Here, the version is specified as a query parameter in the URL.
Example:
– `api/products?version=1`
This approach keeps the URL structure clean but can become cumbersome to manage with extensive API endpoints.
3.3. Header Versioning
Version information is passed in the header of the HTTP request.
Example:
– `Accept: application/vnd.company.app-v1+json`
This method is less intrusive and keeps URLs clean, but it requires clients to modify request headers, which might not always be ideal.
3.4. Hostname Versioning
Different versions are hosted on different subdomains.
Example:
– Version 1: `v1.api.company.com`
– Version 2: `v2.api.company.com`
While this can be clear and systematic, it might complicate hosting and SSL certification.
4. Implementing Versioning in Django
Django REST framework (DRF) provides built-in support for API versioning. Here’s a basic guide to implementing versioning:
4.1. Setting up Django REST Framework
First, ensure that Django and DRF are installed in your project. You can find detailed installation instructions on the Django and DRF official websites (insert relevant links here).
4.2. Configuring Versioning in Django
In `settings.py`, configure the versioning scheme:
```python REST_FRAMEWORK = { 'DEFAULT_VERSIONING_CLASS': 'rest_framework.versioning.URLPathVersioning', 'DEFAULT_VERSION': 'v1', 'ALLOWED_VERSIONS': ['v1', 'v2'], } ```
4.3. Creating Versioned Views
In your views, you can access the version through `request.version`. Use this to write conditional logic based on the API version.
4.4. Migrating Data for New Versions
When introducing a new version, ensure that your database schema and data are compatible. Django’s migration system can help with this process. (Link to a Django migration guide here).
5. Challenges and Best Practices
5.1. Ensuring Consistent Documentation
As you version your API, it’s crucial to keep your documentation up to date. Tools like Swagger or Redoc can help automate this process. (Insert link to tools here).
5.2. Deprecation Strategy
Clearly communicate the deprecation of old API versions to your users. Set a reasonable timeline for clients to migrate to the newer version.
5.3. Testing Across Versions
Ensure comprehensive testing across all API versions. Automated testing can be a lifesaver here.
Read more: MDN Web Docs
Conclusion
API versioning in Django is a critical aspect of developing scalable and robust web applications. By choosing the right versioning strategy and following best practices, you can ensure a smooth transition for your users and maintain a stable API service.
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Django Real-time Alerts: A Push Notification Guide along with the Master Real-Time Communication in Django Apps with WebSockets and The Essential Guide to Social Logins in Django with OAuth and OpenID which will help you understand and gain more insight into the Django programming language.
Table of Contents
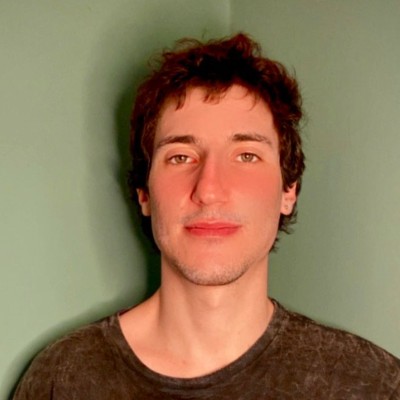
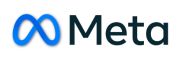