Transforming Web Development: Building Powerful APIs with Django REST Framework
The Django REST Framework (DRF) has become a prominent choice among developers, which includes many businesses seeking to hire Django developers, who aspire to build robust, scalable, and sophisticated APIs. Its powerful toolkit and flexible customization options make it an excellent choice for API development, especially when paired with Django’s ability to handle complex web development tasks.
This demand to hire Django developers also stems from the fact that they are well-equipped to fully harness the capabilities of the DRF. This post aims to delve into the practicalities of using the DRF for creating APIs, a task frequently undertaken by expert Django developers. We’ll be creating a simple API that performs CRUD (Create, Retrieve, Update, Delete) operations, a basic yet fundamental aspect that any Django developer would master.
Introduction to Django REST Framework
The Django REST Framework is a flexible toolkit for building Web APIs. It’s a modular, customizable, and adaptable system that builds on the core concepts of Django to help developers create APIs. It offers advanced features like serialization, query parameter processing, pagination, and more.
Setting Up Django REST Framework
Before we delve into building APIs, you should set up the Django REST Framework. Here’s a brief guide to installing and setting up Django and the REST Framework:
- Create a virtual environment: `python3 -m venv tutorial-env`
- Activate the virtual environment: `source tutorial-env/bin/activate`
- Install Django and Django REST Framework: `pip install django djangorestframework`
- Create a new Django project: `django-admin startproject api_project`
- Navigate into your project: `cd api_project`
- Create a new Django application: `python manage.py startapp blog`
Don’t forget to add `‘rest_framework’` and `’blog’` to your `INSTALLED_APPS` setting.
Creating Models
For our blog API, we will have a simple `Post` model. In your `blog/models.py`, add the following:
```python from django.db import models class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() author = models.CharField(max_length=100) created_at = models.DateTimeField(auto_now_add=True) def __str__(self): return self.title ```
Remember to apply these changes to the database using the commands:
- 1. `python manage.py makemigrations blog`
- 2. `python manage.py migrate`
Serializers
Serializers allow complex data such as querysets and model instances to be converted to native Python data types that can then be easily rendered into JSON, XML, or other content types. In the file `blog/serializers.py`, add:
```python from rest_framework import serializers from .models import Post class PostSerializer(serializers.ModelSerializer): class Meta: model = Post fields = ['id', 'title', 'content', 'author', 'created_at'] ```
Views and APIViews
For our API, we will use Django REST Framework’s APIViews. Create a file `blog/views.py`, and add:
```python from rest_framework import generics from .models import Post from .serializers import PostSerializer class PostList(generics.ListCreateAPIView): queryset = Post.objects.all() serializer_class = PostSerializer class PostDetail(generics.RetrieveUpdateDestroyAPIView): queryset = Post.objects.all() serializer_class = PostSerializer ```
Here, `PostList` handles the `GET` and `POST` requests, while `PostDetail` takes care of `GET`, `PUT`, `PATCH`, and `DELETE` for individual posts.
URL Configuration
Next, define URL patterns for the views. In the file `blog/urls.py`, add:
```python from django.urls import path from .views import PostList, PostDetail urlpatterns = [ path('<int:pk>/', PostDetail.as_view(), name='post_detail'), path('', PostList.as_view(), name='post_list'), ] ```
In the project level `urls.py` (i.e., `api_project/urls.py`), include the app URLs:
```python from django.urls import path, include urlpatterns = [ path('blog/', include('blog.urls')), ] ```
Testing the API
With everything in place, you can now run your server: `python manage.py runserver`
Using a tool like curl or HTTPie, you can test your API:
Create a new post:
``` http POST http://127.0.0.1:8000/blog/ title="My First Post" content="This is the content of my first post" author="John Doe" ```
Retrieve all posts:
``` http GET http://127.0.0.1:8000/blog/ ```
Update a post:
``` http PUT http://127.0.0.1:8000/blog/1/ title="My Updated First Post" ```
Delete a post:
``` http DELETE http://127.0.0.1:8000/blog/1/ ```
Conclusion
In this article, we learned how to set up the Django REST Framework, create a simple API, and test it. The DRF is a powerful toolset that makes it ideal for modern web API development, and its proficiency is a key reason to hire Django developers for your projects.
These examples barely scratch the surface of what’s possible with DRF, but they provide a solid foundation for building more complex APIs. Experienced Django developers can leverage the full potential of these tools in diverse and intricate projects.
Remember, when building an API, focus on creating a robust, clean, and easily maintainable structure. Hiring skilled Django developers can ensure this, bringing deep knowledge of the Django REST Framework’s built-in features, like pagination and authentication, to build secure and user-friendly APIs. Happy coding!
Table of Contents
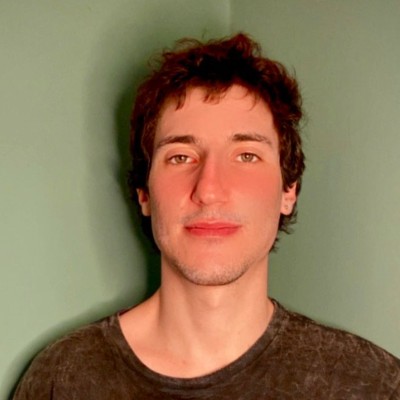
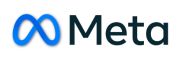