How do I create and apply custom database indexes in Django?
Creating and applying custom database indexes in Django allows you to optimize query performance for specific database queries. Indexes are data structures that improve the speed of data retrieval operations by creating a sorted list of values in one or more columns of a database table. Here’s how you can create and apply custom database indexes in Django:
Create Custom Database Indexes:
- Define the Index: In your Django model, you can define custom indexes by using the `indexes` option within the model’s `Meta` class. For example, if you have a `Book` model and want to create an index on the `title` field, you can do it like this:
```python class Book(models.Model): title = models.CharField(max_length=100) author = models.CharField(max_length=100) class Meta: indexes = [ models.Index(fields=['title']) ] ```
In this example, we define an index on the `title` field.
- Specify Index Type (Optional): You can also specify the index type (e.g., B-tree, Hash, Gist) using the `type` parameter. For example:
```python class Meta: indexes = [ models.Index(fields=['title'], name='book_title_idx', type='btree') ] ```
Django supports different database backends, and the available index types may vary depending on the database you’re using.
Apply Custom Database Indexes:
- Create Migrations: After defining the custom indexes in your model, create a migration using the `makemigrations` command:
``` python manage.py makemigrations ```
This command generates a migration file that includes the instructions for creating the custom index.
- Apply Migrations: Apply the migration to create the custom index in the database:
``` python manage.py migrate ```
Django will execute the necessary SQL statements to create the index in the database.
Custom database indexes can significantly improve query performance for fields that are frequently used in filtering or sorting operations. However, it’s important to use indexes judiciously, as they can increase storage requirements and have a small impact on insert and update operations. Monitoring and optimizing database performance is an ongoing process, and custom indexes can be a valuable tool in your toolbox for achieving this.
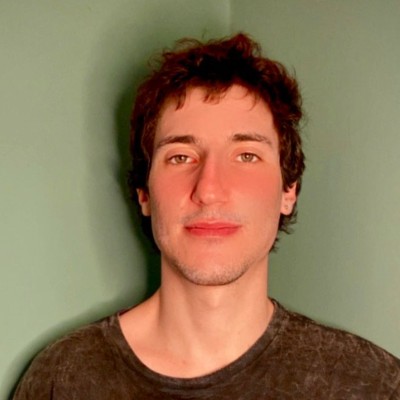
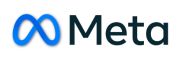