Django Authentication: Securing User Accounts in Your Web App
In the digital age, the importance of creating secure web applications cannot be understated. This holds especially true when dealing with user accounts, where sensitive data is often at stake. Companies often hire Django developers to safeguard this sensitive information. Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design, provides powerful tools that these developers can use to protect user data. Today, we’ll delve into Django Authentication — a robust built-in system that ensures the security of user accounts in your web app. This functionality is part of why businesses choose to hire Django developers for their projects.
What is Django Authentication?
Django comes with a built-in system for handling user authentication. This includes user accounts, user roles, permissions, and sessions. It’s a highly secure, flexible, and powerful tool. Django’s authentication system handles both authentication (verifying who you are) and authorization (what you are allowed to do).
Setting Up Django Authentication
Before we can implement authentication, we need a Django project to work on. If you aren’t familiar with Django, you might consider the option to hire Django developers to expedite this process. If you’re taking on the task yourself, you’ll need to install Django, set up a project, and create an application. Django’s official documentation offers a comprehensive guide on setting up your environment. After setting up, your directory structure should look something like this. Remember, if this seems daunting, you can always hire Django developers to ensure a smooth and secure setup.
myproject/ manage.py myproject/ __init__.py settings.py urls.py asgi.py wsgi.py myapp/ __init__.py admin.py apps.py migrations/ __init__.py models.py tests.py views.py
Building User Model and Forms
After setting up your environment, let’s create a User model and registration form. Fortunately, Django provides a built-in `User` model which you can import using `from django.contrib.auth.models import User`. You can also use `UserCreationForm` that Django provides by default.
However, if you need a custom user model with additional fields, Django allows you to extend its built-in user model:
```python from django.contrib.auth.models import AbstractUser class CustomUser(AbstractUser): phone_number = models.CharField(max_length=20, null=True, blank=True)
Next, let’s create a User Registration Form:
```python from django import forms from django.contrib.auth.forms import UserCreationForm from .models import CustomUser class NewUserForm(UserCreationForm): class Meta: model = CustomUser fields = ("username", "email", "password1", "password2", "phone_number")
Handling User Registration
Now we’ve defined our User model and registration form, let’s create a view to handle user registration.
```python from django.shortcuts import render, redirect from .forms import NewUserForm from django.contrib import messages def register_request(request): if request.method == "POST": form = NewUserForm(request.POST) if form.is_valid(): user = form.save() messages.success(request, "Registration successful." ) return redirect("myapp:login") messages.error(request, "Unsuccessful registration. Invalid information.") form = NewUserForm() return render (request=request, template_name="myapp/register.html", context={"register_form":form})
In this code, we’re creating a `register_request` view that accepts both GET and POST requests. For a POST request, it attempts to validate and save the form data. If successful, it redirects the user to the login page; otherwise, it returns an error message. For a GET request, it simply displays an empty registration form.
Implementing User Login
For user login, Django provides a built-in `AuthenticationForm`. We can use it in our login view
```python from django.contrib.auth import login from django.contrib.auth.forms import AuthenticationForm def login_request(request): if request.method == "POST": form = AuthenticationForm(request, data=request.POST) if form.is_valid(): username = form.cleaned_data.get('username') password = form.cleaned_data.get('password') user = authenticate(username=username, password=password) if user is not None: login(request, user) messages.info(request, f"You are now logged in as {username}.") return redirect("myapp:main") else: messages.error(request,"Invalid username or password.") else: messages.error(request,"Invalid username or password.") form = AuthenticationForm() return render(request=request, template_name="myapp/login.html", context={"login_form":form})
In this code, we are creating a `login_request` view that, similar to our registration view, accepts both GET and POST requests. For a POST request, it validates the form data, authenticates the user, logs them in, and redirects them to the main page. If the form data is invalid or authentication fails, it returns an error message. For a GET request, it simply displays the empty login form.
Conclusion
Through Django’s Authentication system, developers can build secure, robust applications while focusing on their app’s unique features. Django’s built-in User model, forms, and views allow you to handle user registration, login, and session management with minimal setup. These features are why many businesses choose to hire Django developers when they need to ensure their web applications are secure and efficient.
However, this only scratches the surface of what you can achieve with Django’s authentication system. For more advanced functionality, such as password resets, user permissions, and custom user models, you may want to refer to Django’s comprehensive authentication documentation or consider hiring Django developers for their expertise. Remember, the security of your users’ data is paramount, so always keep up to date with Django’s best practices. If this becomes overwhelming, don’t hesitate to hire Django developers. They can help secure your web app while allowing you to focus on its core functionality.
Table of Contents
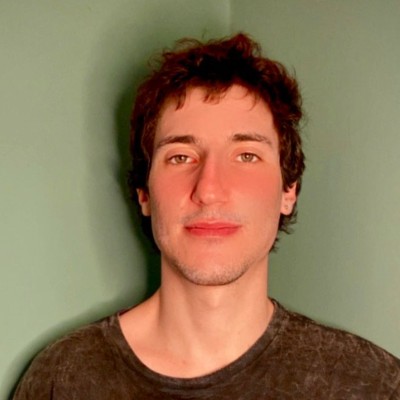
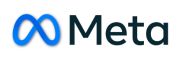