Django Q & A
How to use Django’s authentication system?
Django’s authentication system is a built-in feature that simplifies user management and authentication in web applications. It provides a robust and secure way to handle user registration, login, password management, and user sessions. Here’s a guide on how to use Django’s authentication system effectively:
- Setting Up Authentication: To use Django’s authentication, start by ensuring it’s included in your project’s settings. Open your project’s `settings.py` file and find the `INSTALLED_APPS` section. Make sure `’django.contrib.auth’` and `’django.contrib.contenttypes’` are included.
- User Model: Django’s authentication system comes with a built-in User model, but you can also create your own custom User model by extending the `AbstractUser` class. This allows you to add custom fields to the User model to store additional user information.
- User Registration: To allow users to register, create a registration form and view. The form should extend `UserCreationForm` from `django.contrib.auth.forms`, and the view should handle form validation and user creation. After successful registration, redirect the user to a login page or automatically log them in.
- User Login: Create a login form and view using `AuthenticationForm` from `django.contrib.auth.forms`. In the view, handle user login and session management. Upon successful login, you can redirect the user to a dashboard or any authenticated area.
- Authentication Middleware: Django’s authentication middleware helps manage user sessions and secure views. Ensure that the `AuthenticationMiddleware` is included in your `MIDDLEWARE` setting in `settings.py`.
- User Authentication in Views: To protect specific views or resources, use the `@login_required` decorator, which ensures that only authenticated users can access those views. You can apply this decorator to individual views or to an entire URL pattern in your `urls.py`.
- Password Reset: Django provides built-in views and templates for password reset functionality. You can include these views in your project’s `urls.py` and customize the templates to match your application’s design.
- User Management: Administrators can manage users, including creating, updating, and deleting user accounts, through the Django admin panel. Ensure that the `django.contrib.auth.admin` app is included in your `INSTALLED_APPS`.
- Custom User Authentication: If your application requires custom authentication methods or integration with third-party authentication providers (e.g., OAuth), Django allows you to extend or replace its authentication system to meet your specific needs.
By following these steps, you can effectively use Django’s authentication system to handle user registration, login, and session management in your web application. Django’s built-in tools and flexibility make it a robust choice for handling user authentication securely and efficiently.
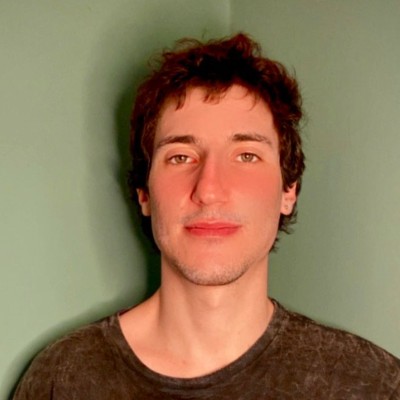
Previously at
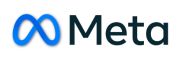
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.