Django for Beginners: Getting Started with Web Development
If you’re just beginning your journey into web development, Django is an excellent choice for a framework to learn. Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design, making it a popular choice for businesses looking to hire Django developers. It follows the “batteries included” philosophy, meaning it comes with everything you need to build a web application out-of-the-box. Whether you’re a business looking to hire Django developers or a beginner diving into web development, this article will provide a detailed introduction to Django, helping you get started with this powerful tool.
What is Django?
Django is a free and open-source web framework written in Python. It follows the Model-View-Controller (MVC) architectural pattern and is designed to help developers create complex, database-driven websites with ease. Django emphasizes reusability and “pluggability” of components, less code, low coupling, and the principle of “don’t repeat yourself” (DRY). With Django, you can build a wide variety of applications ranging from simple websites to complex, data-driven web applications.
Setting Up Django
Before you begin with Django, which is a preferred framework for many businesses looking to hire Django developers, you’ll need to have Python installed on your system. Django works with Python 3.6 and newer versions. To check if you have Python installed, open a terminal and run:
```bash python --version
Once you’ve confirmed your Python installation, you can install Django by using pip, the Python package installer. Run the following command in your terminal:
```bash pip install Django
To verify that Django was installed successfully, you can do this by running:
```bash python -m django --version
Creating Your First Django Project
Now that Django is installed, you’re ready to create your first Django project. A project in Django is essentially a collection of settings and configurations for a specific website, which can contain multiple applications. To create a new project, navigate to the directory where you want your project to be, and run:
```bash django-admin startproject myfirstproject
This will create a new Django project called ‘myfirstproject’. Change into the new project directory with:
```bash cd myfirstproject
In this directory, you’ll find manage.py (a command-line utility that lets you interact with Django in various ways) and a myfirstproject directory (the actual project package).
Running the Development Server
Django comes with a lightweight web server for development. This is not meant for production use, but it’s perfect for our learning purposes. You can start the server with the following command:
```bash python manage.py runserver
If everything is working correctly, you should see a message stating that the development server is running at http://127.0.0.1:8000/. If you navigate to this URL in your web browser, you should see a welcome page confirming that Django is working.
Creating a Django Application
A Django project can consist of multiple apps, each serving a specific function. For example, a blogging platform might have separate apps for handling user authentication, blog posts, comments, etc. To create an app within your project, run:
```bash python manage.py startapp myfirstapp
This will create a directory ‘myfirstapp’, which includes several files for handling the various aspects of your application, like database models, views, templates, etc.
Understanding Django’s MVT Architecture
Django follows a slightly different take on the traditional MVC pattern, known as the Model-View-Template (MVT) pattern:
- Model: This is the data access layer. It handles everything related to the data: how to access it, how to validate it, what behaviors it has, and the relationships between the data.
- View: The view is the business logic layer. It controls the flow of information between the models and templates.
- Template: This is the presentation layer. It deals with how the data gets presented to the user. Django’s template language is designed to be easy to use while maintaining the power of Python.
Building Your First View
Let’s create a simple view that returns a “Hello, Django!” message. In myfirstapp/views.py, add the following code:
```python from django.http import HttpResponse from django.shortcuts import render def hello(request): return HttpResponse("Hello, Django!")
This function, `hello`, is the simplest view possible in Django. To call the view, we need to map it to a URL – and for this, we need a URLconf.
In the myfirstapp directory, create a file called urls.py. Your app directory should now look like:
myfirstapp/ __init__.py admin.py apps.py migrations/ __init__.py models.py tests.py views.py urls.py
In the myfirstapp/urls.py file include the following code:
```python from django.urls import path from . import views urlpatterns = [ path('', views.hello, name='hello'),
The next step is to point the root URLconf at the myfirstapp.urls module. In myfirstproject/urls.py, add an import for django.urls.include and insert an include() in the urlpatterns list, so you have:
```python from django.contrib import admin from django.urls import include, path urlpatterns = [ path('myfirstapp/', include('myfirstapp.urls')), path('admin/', admin.site.urls), ]
You have now wired an index view into the URLconf. Verify it’s working with `python manage.py runserver`. Go to http://localhost:8000/myfirstapp in your browser, and you should see the text “Hello, Django!”, which you defined in the hello view.
Conclusion
We’ve just scratched the surface of Django, but you should now have a good understanding of what Django is, how to install it, create a project, run a development server, create an app, and build a simple view. As you acquire these skills, you not only grow as a developer but also become more appealing to businesses that are looking to hire Django developers.
The next steps could be learning about Django models and databases, forms, user authentication, testing, and deploying your application to a live server. Such knowledge is key, whether you aspire to develop your own applications or seek opportunities to get hired as a Django developer. Django has a great community and a wealth of resources available online, so don’t hesitate to explore and experiment. Happy coding!
Table of Contents
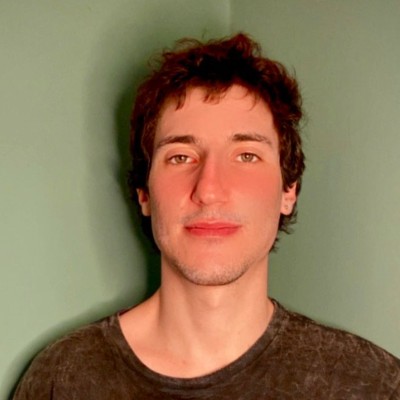
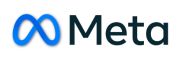