Django Q & A
How to use built-in authentication with Django third-party login providers (OAuth)?
Integrating Django’s built-in authentication with third-party login providers using OAuth is a common and powerful feature that allows users to log in or sign up to your Django web application using their existing accounts from providers like Google, Facebook, or GitHub. Here’s how to implement this functionality:
- Install Required Packages: To get started, you’ll need to install packages that facilitate OAuth integration. The most commonly used package is `django-allauth`. You can install it using pip:
``` pip install django-allauth ```
- Add to Installed Apps: In your project’s `settings.py`, add `’allauth’` and `’allauth.account’` to the `INSTALLED_APPS` list.
- Configuration: Configure the authentication settings in `settings.py`. For example, you can set the authentication backend and the redirect URL after login:
```python AUTHENTICATION_BACKENDS = ( ... 'allauth.account.auth_backends.AuthenticationBackend', ) LOGIN_REDIRECT_URL = '/' ```
- Provider Setup: Configure OAuth providers in your project settings. For example, to enable Google OAuth, you’ll need to set up the OAuth keys and secrets provided by Google in your settings:
```python SOCIALACCOUNT_PROVIDERS = { 'google': { 'APP': { 'client_id': 'your-client-id', 'secret': 'your-secret-key', 'key': '' } } } ```
- URLs Configuration: Include the necessary URLs for authentication in your project’s `urls.py`:
```python path('accounts/', include('allauth.urls')), path('accounts/', include('allauth.socialaccount.urls')), ```
- Templates and Views: Customize templates and views as needed. You can override the default templates provided by `allauth` to match your application’s design and branding.
- Testing and Debugging: Thoroughly test the authentication flow, ensuring that users can log in and sign up using third-party OAuth providers. Use Django’s built-in debugging tools to troubleshoot any issues.
By following these steps and referring to the documentation of the OAuth provider you want to integrate (e.g., Google, Facebook), you can enable third-party authentication in your Django application, enhancing user convenience and expanding your user base by allowing users to log in using their preferred social media or OAuth accounts.
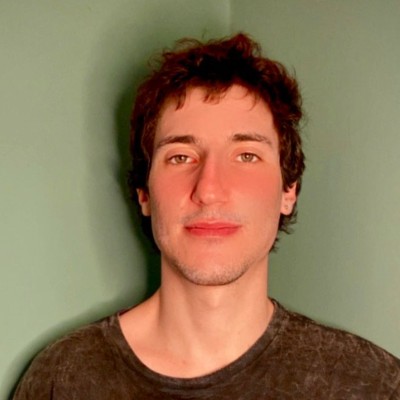
Previously at
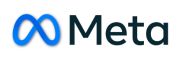
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.