How to create and manage user sessions in Django?
Creating and managing user sessions in Django is a fundamental aspect of web application development, especially when dealing with user authentication and user-specific data. Django provides a robust session framework that simplifies session management. Here’s how to create and manage user sessions in Django:
- Enabling Sessions:
By default, Django’s session framework is enabled. To ensure it’s active in your project, make sure the `django.contrib.sessions.middleware.SessionMiddleware` is included in your `MIDDLEWARE` setting in your project’s settings.py file.
- Session Configuration:
In your settings.py file, you can configure various aspects of sessions, such as the session engine, session expiration, and more. The default session engine uses Django’s database to store session data, but you can choose other options like using caches or a database-backed cache.
- Storing Data in Sessions:
To store data in a user’s session, you can use the `request.session` object, which is a dictionary-like object. For example, to store a user’s username:
```python request.session['username'] = 'example_user' ```
- Retrieving Data from Sessions:
You can retrieve data from sessions by accessing the `request.session` object. For example, to get the stored username:
```python username = request.session.get('username', 'default_value') ```
- Clearing Data from Sessions:
To remove data from a session, you can use the `del` statement or the `pop` method. For example:
```python del request.session['username'] # or request.session.pop('username', None) ```
- Session Expiration:
Django allows you to set session expiration policies. You can define how long a session should last before it expires, or it can expire when the user closes their browser (a session cookie).
- Logout and Session Cleanup:
When a user logs out, it’s essential to clear their session data to ensure their previous session data is not accessible. Django provides a `django.contrib.auth.logout` function that takes care of this for you.
By following these steps, you can create and manage user sessions in Django effectively. Django’s built-in session framework provides a secure and convenient way to handle user-specific data and authentication in your web applications.
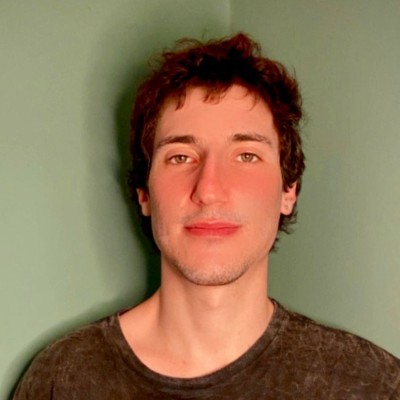
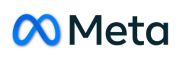