How to create custom Django management commands?
Creating custom Django management commands is a useful way to automate tasks specific to your Django project, making it easier to perform routine operations, data migrations, or other custom actions. Here’s a step-by-step guide on how to create custom Django management commands:
- Create a Management Command File:
In your Django app, create a Python file within a `management/commands` directory. The directory structure should look like this:
``` your_app/ ??? management/ ? ??? commands/ ? ??? my_custom_command.py ```
- Define the Command:
In `my_custom_command.py`, define your custom management command by creating a Python class that inherits from `django.core.management.base.BaseCommand`. You need to implement the `handle` method, which contains the logic of your command. Here’s a minimal example:
```python from django.core.management.base import BaseCommand class Command(BaseCommand): help = 'My custom Django management command' def handle(self, *args, **options): self.stdout.write(self.style.SUCCESS('Successfully executed my custom command')) ```
- Implement Your Command Logic:
Within the `handle` method, write the logic for your custom command. You can perform database operations, make API requests, generate reports, or any other task specific to your project.
- Test Your Custom Command:
Before using the custom command in production, test it locally by running:
```bash python manage.py my_custom_command ```
Replace `my_custom_command` with the name of your custom command.
- Document Your Command:
It’s essential to provide clear documentation for your custom command, including its purpose, usage, and any required arguments or options. You can add a docstring to the command class and include usage instructions.
- Add the Command to Your Project’s Documentation:
If your project has documentation, make sure to include information about your custom command so that other developers can use it effectively.
- Version Control:
Don’t forget to commit your custom management command to your version control system, ensuring that it’s part of your project’s codebase.
By creating custom Django management commands, you can automate repetitive tasks, streamline your development process, and make it easier for your team to maintain and manage the project. These commands become invaluable tools as your project grows and requires more complex operations.
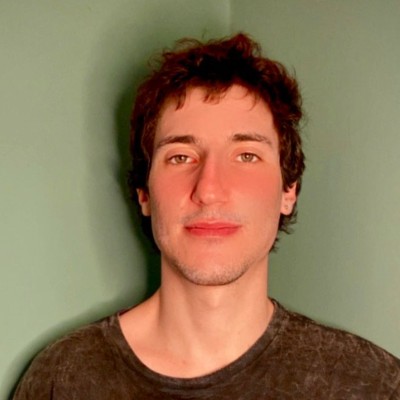
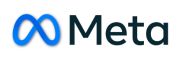