How can I create a custom user model in Django?
Creating a custom user model in Django is a common requirement when you need to extend the default `User` model provided by Django’s authentication system. Custom user models allow you to add additional fields and behaviors to user profiles. Here’s a step-by-step guide on how to create a custom user model in Django:
- Create a New Django App:
To keep your custom user model separate from other models, create a new Django app. You can name it something like `accounts` or `users`.
```bash python manage.py startapp accounts ```
- Define Your Custom User Model:
In the `models.py` file of your new app, create your custom user model by subclassing `AbstractBaseUser` and `PermissionsMixin` from Django’s `auth` module. Define any additional fields you need for your user model.
```python from django.contrib.auth.models import AbstractBaseUser, BaseUserManager, PermissionsMixin from django.db import models class CustomUserManager(BaseUserManager): def create_user(self, email, password=None, **extra_fields): # Create and save a new user with the given email and password if not email: raise ValueError('The Email field must be set') email = self.normalize_email(email) user = self.model(email=email, **extra_fields) user.set_password(password) user.save(using=self._db) return user def create_superuser(self, email, password=None, **extra_fields): # Create and save a new superuser with the given email and password extra_fields.setdefault('is_staff', True) extra_fields.setdefault('is_superuser', True) if extra_fields.get('is_staff') is not True: raise ValueError('Superuser must have is_staff=True.') if extra_fields.get('is_superuser') is not True: raise ValueError('Superuser must have is_superuser=True.') return self.create_user(email, password, **extra_fields) class CustomUser(AbstractBaseUser, PermissionsMixin): email = models.EmailField(unique=True) first_name = models.CharField(max_length=30) last_name = models.CharField(max_length=30) is_active = models.BooleanField(default=True) is_staff = models.BooleanField(default=False) date_joined = models.DateTimeField(auto_now_add=True) objects = CustomUserManager() USERNAME_FIELD = 'email' REQUIRED_FIELDS = [] def __str__(self): return self.email ```
- Update Your Project’s Settings:
In your project’s settings (usually in `settings.py`), set the `AUTH_USER_MODEL` to point to your custom user model:
```python AUTH_USER_MODEL = 'accounts.CustomUser' ```
- Create and Apply Migrations:
Generate and apply database migrations to create the new user model table:
```bash python manage.py makemigrations python manage.py migrate ```
- Update References:
Search your project for any references to the old user model and update them to use your custom user model. This includes any foreign keys or many-to-many relationships.
By following these steps, you’ll have successfully created a custom user model in Django, allowing you to tailor the user authentication system to your application’s specific needs. Your custom user model can include any additional fields and methods you require for your project.
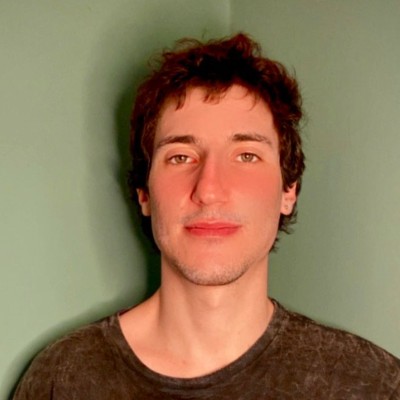
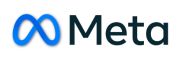