From Raw Data to Visual Storytelling: Django Data Analytics Uncovered
The power of web applications lies not only in their ability to facilitate user engagement and perform actions but also in their capability to gather and analyze data. Django, a high-level Python web framework, provides tools that can be integrated with various data analytics tools to gain insights from the data. In this blog post, we will explore how to use Django with data analytics, illustrated with examples.
Table of Contents
1. The Intersection of Django and Analytics
Django is a powerful framework for building web applications quickly, while maintaining the flexibility and scalability to handle complex projects. One of its strongest features is the ORM (Object-Relational Mapping) which abstracts database operations and helps in data modeling. This data can be a goldmine for insights, and data analytics tools can help unearth these insights.
2. Setting up Django Models for Analytics
The foundation of data analytics in Django starts with the model. Properly designed models ensure that data is stored efficiently and can be retrieved for analysis easily.
Example:
Consider a simple e-commerce application. One might have a model for Orders as:
```python from django.db import models class Order(models.Model): user = models.ForeignKey(User, on_delete=models.CASCADE) product = models.ForeignKey(Product, on_delete=models.CASCADE) date_ordered = models.DateTimeField(auto_now_add=True) quantity = models.PositiveIntegerField() total_price = models.DecimalField(max_digits=10, decimal_places=2) ```
3. Extracting Basic Insights with Django ORM
With Django ORM, you can fetch, filter, and aggregate data directly from the database.
Example:
To get the total sales for a particular product:
```python total_sales = Order.objects.filter(product=specific_product).aggregate(total=models.Sum('total_price'))['total'] ```
4. Integrating Django with Data Visualization Tools
While raw data and numbers are great, visualization tools like Matplotlib, Seaborn, or Plotly can provide insights visually.
Example:
Plotting monthly sales:
```python import matplotlib.pyplot as plt from django.db.models.functions import TruncMonth from django.db.models import Sum data = Order.objects.annotate(month=TruncMonth('date_ordered')).values('month').annotate(total_sales=Sum('total_price')) months = [item['month'] for item in data] sales = [item['total_sales'] for item in data] plt.plot(months, sales) plt.title('Monthly Sales') plt.xlabel('Month') plt.ylabel('Total Sales') plt.show() ```
5. Advanced Analytics with Pandas and Django
Pandas is a powerful data analysis tool that can work smoothly with Django QuerySets.
Example:
Getting the average order value by user:
```python import pandas as pd orders = Order.objects.all().values() df = pd.DataFrame.from_records(orders) avg_order_value = df.groupby('user').total_price.mean() ```
6. Enhancing Insights with Machine Learning
You can also combine Django with tools like Scikit-learn to apply machine learning on your data for predictive analytics.
Example:
Predicting future sales based on historical data using a simple linear regression:
```python from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression data = Order.objects.annotate(month=TruncMonth('date_ordered')).values('month').annotate(total_sales=Sum('total_price')) df = pd.DataFrame.from_records(data) X = df['month'].values.reshape(-1,1) y = df['total_sales'].values X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) model = LinearRegression().fit(X_train, y_train) future_month = [[new_month]] predicted_sales = model.predict(future_month) ```
Conclusion
Django, with its powerful ORM and the ecosystem of Python libraries, provides an excellent platform for data analytics. Whether you’re simply tallying up metrics, generating visual reports, or diving into advanced predictive analytics, Django can be an integral part of your data-driven journey.
By setting up your Django models optimally, leveraging the ORM for queries, integrating with data visualization tools, and using data science libraries, you can extract significant insights from your app’s data. As your data grows, these insights can be instrumental in steering the direction of your application or business strategy.
Table of Contents
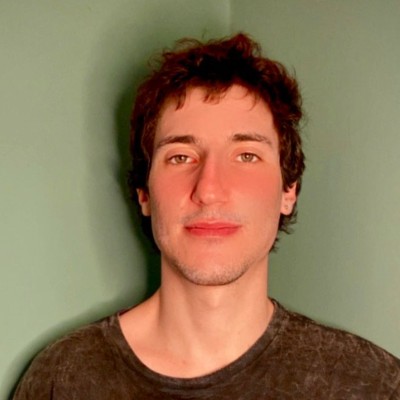
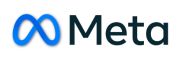