Navigating Data Validation in Django: Tips, Tricks, and Techniques
In the world of web development, data integrity is crucial. Ensuring that the data entering your system is valid, consistent, and secure can make or break the functionality and reputation of your web application. This is where Django, a high-level Python web framework, shines with its robust data validation capabilities. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. Understanding Django’s Data Validation System
Django’s data validation system is built around its models and forms. It provides various tools and techniques to validate data at different levels – from field-specific validators to form-level cleaning methods.
1.1 Field-Level Validation
Every field in a Django model or form can have built-in validators. These are Python functions that take a value and raise a `ValidationError` if the value doesn’t meet certain criteria. For example, the `EmailField` in Django automatically checks if an input is a valid email address.
Example:
```python from django.db import models from django.core.validators import MaxValueValidator, MinValueValidator class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) quantity = models.IntegerField(validators=[MinValueValidator(1), MaxValueValidator(100)]) ```
In this example, the `quantity` field uses `MinValueValidator` and `MaxValueValidator` to ensure its value is between 1 and 100.
1.2 Form-Level Validation
Django forms also offer a higher level of data validation. The `clean()` method allows you to perform custom validations across different fields.
Example:
```python from django import forms class OrderForm(forms.Form): product_id = forms.IntegerField() quantity = forms.IntegerField() def clean(self): cleaned_data = super().clean() quantity = cleaned_data.get("quantity") if quantity < 1: raise forms.ValidationError("Quantity must be at least 1") return cleaned_data ```
Here, the `clean()` method checks if the `quantity` is less than 1 and raises a `ValidationError` if so.
2. Best Practices for Data Validation in Django
To ensure data integrity in your Django web app, follow these best practices:
- Use Django’s Built-in Validators: Leverage Django’s rich set of built-in field validators for common data types and validation scenarios.
- Write Custom Validators When Necessary: If built-in validators are not sufficient, write custom validators for more complex or specific validation logic.
- Validate at Form Level for Complex Logic: Use form-level validation for cross-field validation and more complex validation scenarios.
- Keep Business Logic Separate: Avoid embedding complex business logic in validators. Use Django’s model methods or service layers for this.
- Test Your Validators: Write unit tests for your custom validators to ensure they work as expected.
3. Advanced Techniques in Django Data Validation
3.1 Using Django REST Framework for API Validation
If you’re building a RESTful API with Django, the Django REST Framework (DRF) offers powerful serialization and validation tools. DRF serializers allow for easy and robust request data validation.
Example:
```python from rest_framework import serializers class ProductSerializer(serializers.Serializer): name = serializers.CharField(max_length=100) price = serializers.DecimalField(max_digits=10, decimal_places=2) quantity = serializers.IntegerField(min_value=1, max_value=100) ```
In this serializer, validation is defined similarly to Django forms and model fields.
3.2 Implementing Custom Field Validators
For unique validation rules, you can implement custom field validators.
Example:
```python from django.core.exceptions import ValidationError def validate_positive(value): if value <= 0: raise ValidationError('Value must be positive') class Product(models.Model): stock = models.IntegerField(validators=[validate_positive]) ```
Here, `validate_positive` ensures the `stock` value is always positive.
Conclusion
Django’s comprehensive data validation system is a powerful tool for maintaining data integrity in web applications. By understanding and utilizing Django’s validators, forms, and REST framework serializers, you can ensure that the data in your web app is valid, consistent, and secure.
For more insights on Django and data validation, explore these additional resources:
- Django’s Official Documentation on Validators
- Tutorial on Django Forms and Field Validation
- Django REST Framework Documentation for Serialization and Validation
Remember, effective data validation is key to the reliability and success of your web application.
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Django for Beginners: Getting Started with Web Development along with the Django Admin: A Powerful Tool for Managing Your Web Application and Django Performance Optimization: Boosting Your Web App’s Speed which will help you understand and gain more insight into the Django programming language.
Table of Contents
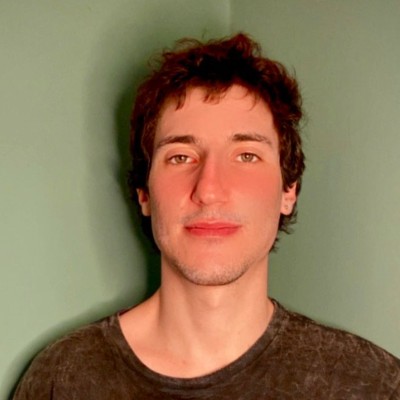
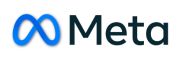