Elasticsearch: The Game-Changer for Your Django App’s Search Function
In an increasingly data-driven world, it’s crucial to ensure that your application’s search functionality is both robust and efficient. One effective strategy to enhance search capabilities is to hire Django developers for the integration of Elasticsearch, a popular, open-source search and analytics engine. When paired with Django, a high-level Python web framework, these skilled professionals can create applications that efficiently handle complex search queries and return relevant results in real time.
Table of Contents
In this blog post, we will delve into how to integrate Elasticsearch with a Django application. Whether you plan to do it yourself or intend to hire Django developers for the task, this step-by-step guide, replete with code examples, will serve as a valuable resource.
1. Introduction to Elasticsearch
Elasticsearch is a distributed, RESTful search and analytics engine that enables you to search and analyze large volumes of data in real time. It is built on Apache Lucene, a high-performance, full-featured text search engine library.
Elasticsearch is often used for log and event data analysis but it is also extremely efficient for full-text search, structured search, and analytics.
2. Prerequisites
Before we start, you should have the following installed on your machine:
- Python 3.6 or newer
- Django 3.2 or newer
- Elasticsearch 7.x
You should also have a basic understanding of Django and Python.
3. Setting up Elasticsearch
First, download and install Elasticsearch from the official [Elastic website] (https://www.elastic.co/downloads/elasticsearch). After installation, you can start Elasticsearch by navigating to the bin directory and executing:
``` ./elasticsearch ```
By default, Elasticsearch runs on port 9200. You can test if it’s working by visiting `http://localhost:9200` in your web browser.
4. Creating the Django Project
Next, we will set up a new Django project and a new app within the project.
- Create a new project named “blog_search” using the following command:
``` django-admin startproject blog_search ```
- Navigate to the project directory and create a new app named “articles”:
``` cd blog_search django-admin startapp articles ```
In this demo, we’ll be creating a simple blog application where users can search for articles.
5. Installing Django Elasticsearch DSL
To integrate Elasticsearch with Django, we’ll use Django Elasticsearch DSL, a package that allows for easy integration between Django and Elasticsearch.
Install it with pip:
``` pip install django-elasticsearch-dsl ```
6. Configuring Django to Use Elasticsearch
To configure Django to use Elasticsearch, add the following to the `settings.py` file:
```python ELASTICSEARCH_DSL = { 'default': { 'hosts': 'localhost:9200' }, } ```
We also need to add ‘django_elasticsearch_dsl‘ and our ‘articles’ app to the `INSTALLED_APPS` list:
```python INSTALLED_APPS = [ ... 'django_elasticsearch_dsl', 'articles', ] ```
7. Building the Django App
Assume that we have a basic `Article` model in the `models.py` file of the `articles` app:
```python from django.db import models class Article(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True) updated_at = models.DateTimeField(auto_now=True) def __str__(self): return self.title ```
We can index this model in Elasticsearch using Django Elasticsearch DSL. In the `documents.py` file in the `articles` app, add the following:
```python from django_elasticsearch_dsl import Document, Index from django_elasticsearch_dsl.registries import registry from .models import Article articles = Index('articles') @registry.register_document class ArticleDocument(Document): class Index: name = 'articles' settings = {'number_of_shards': 1, 'number_of_replicas': 0} class Django: model = Article fields = [ 'title', 'content', ] ```
The `ArticleDocument` class represents the Elasticsearch document. This class has a nested `Django` class where you specify the Django model to be indexed and a list of fields you want to index.
8. Synchronizing Django and Elasticsearch Data
To keep our Django and Elasticsearch data synchronized, we need to use Django signals. In the `signals.py` file of the `articles` app, add:
```python from django.dispatch import receiver from django.db.models.signals import pre_save from django_elasticsearch_dsl.registries import registry from .models import Article @receiver(pre_save, sender=Article) def update_document(sender, **kwargs): instance = kwargs['instance'] registry.update(instance) ```
Don’t forget to import and include this signal module in your `apps.py`:
```python from django.apps import AppConfig class ArticlesConfig(AppConfig): default_auto_field = 'django.db.models.BigAutoField' name = 'articles' def ready(self): import articles.signals # noqa ```
9. Searching with Elasticsearch
Now that we have set up Elasticsearch and our Django models, we can use Elasticsearch to search our data. In the `views.py` file in the `articles` app, add:
```python from django.shortcuts import render from django_elasticsearch_dsl_drf.viewsets import DocumentViewSet from django_elasticsearch_dsl_drf.filter_backends import ( FilteringFilterBackend, OrderingFilterBackend, DefaultOrderingFilterBackend, SearchFilterBackend, ) from elasticsearch_dsl import Q from .documents import ArticleDocument from .serializers import ArticleDocumentSimpleSerializer class ArticleDocumentView(DocumentViewSet): document = ArticleDocument serializer_class = ArticleDocumentSimpleSerializer lookup_field = 'id' filter_backends = [ FilteringFilterBackend, OrderingFilterBackend, DefaultOrderingFilterBackend, SearchFilterBackend, ] search_fields = ( 'title', 'content', ) filter_fields = { 'title': 'title.raw', 'content': 'content.raw', } ordering_fields = { 'title': 'title.raw', } ordering = ('_score', 'title', 'id',) ```
Here, we create a view that allows us to search our Elasticsearch index. We specify our document, the fields we can search on, the fields we can filter on, and the default ordering of results.
In the `serializers.py` file, add:
```python from django_elasticsearch_dsl_drf.serializers import DocumentSerializer from .documents import ArticleDocument class ArticleDocumentSimpleSerializer(DocumentSerializer): class Meta: document = ArticleDocument fields = ( 'title', 'content', 'id', 'created_at', 'updated_at', ) ```
This serializer tells Django how to return the data.
Wrapping Up
In this guide, we’ve covered the basics of integrating Elasticsearch with a Django application. Whether you choose to leverage these insights yourself or hire Django developers to expedite the process, this foundation will allow you to further explore the vast capabilities of Elasticsearch. The goal is to provide efficient, real-time search functionality for your applications.
Remember, the process we covered here is just the beginning. Elasticsearch offers numerous features and capabilities to analyze and manipulate your data to meet your specific needs. Whether you’re an aspiring coder or a business owner looking to hire Django developers, the road to enhanced search functionality begins here.
Table of Contents
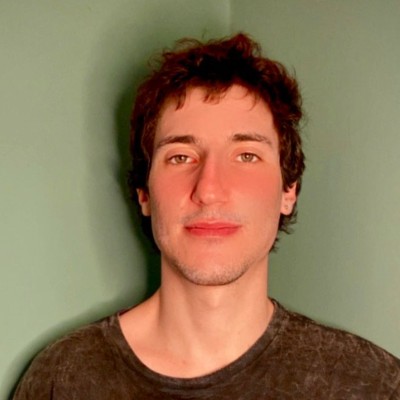
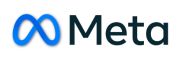