How to handle file uploads in Django using cloud storage like AWS S3?
Handling file uploads in Django using cloud storage services like AWS S3 is a common practice for scalable and reliable storage of user-generated content, such as images, videos, and documents. Here’s a step-by-step guide on how to achieve this:
- Setup AWS S3 Account:
Start by creating an AWS S3 account if you don’t have one already. This service allows you to store and serve files securely in the cloud.
- Install Required Packages:
In your Django project, you’ll need to install the `boto3` library, which is the official AWS SDK for Python, and `django-storages`, a Django library that simplifies working with cloud storage services.
```bash pip install boto3 django-storages ```
- Configure AWS Credentials:
Configure AWS credentials by either setting environment variables or using AWS CLI. Ensure that your IAM (Identity and Access Management) user has the necessary permissions to access S3 buckets.
- Update Django Settings:
In your Django project’s settings, configure the storage backend to use AWS S3 by adding the following code:
```python AWS_ACCESS_KEY_ID = 'your-access-key' AWS_SECRET_ACCESS_KEY = 'your-secret-key' AWS_STORAGE_BUCKET_NAME = 'your-bucket-name' AWS_S3_REGION_NAME = 'your-region' # e.g., 'us-east-1' AWS_S3_CUSTOM_DOMAIN = f'{AWS_STORAGE_BUCKET_NAME}.s3.amazonaws.com' DEFAULT_FILE_STORAGE = 'storages.backends.s3boto3.S3Boto3Storage' ```
- Update Your Model:
Modify your model to include a `FileField` or `ImageField` to handle file uploads. For example:
```python from django.db import models class MyModel(models.Model): uploaded_file = models.FileField(upload_to='uploads/') ```
- Update Your Form and View:
If you’re using forms and views to handle file uploads, ensure they are configured to work with the file fields. In your view, use the `request.FILES` object to access uploaded files.
- Collect Static Files:
If you’re serving static files directly from S3 (e.g., user avatars), use Django’s `collectstatic` management command to upload your static files to S3:
```bash python manage.py collectstatic ```
- Testing and Deployment:
Thoroughly test your file uploads in a development environment before deploying to production. Ensure that your production environment is configured to use S3 for file storage.
By following these steps, you can seamlessly handle file uploads in Django using AWS S3, providing a reliable and scalable solution for storing and serving user-generated content in your web application.
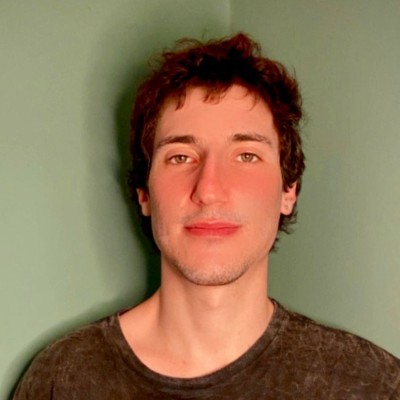
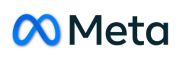