Django Forms: Building User-Friendly Input Mechanisms
Web applications are a cornerstone of our digital society, powering everything from social networks to banking systems. One of the fundamental aspects of these applications is user interaction, which often comes in the form of input fields. These are places where users can input data for the application to process and store. Given their importance, it’s no surprise that businesses often hire Django developers to streamline this process.
In the world of Python web development, Django is a robust and versatile framework, recognized for its simplicity and efficiency. It offers Django developers a powerful tool to handle user interactions: Django Forms. Skilled in creating user-friendly mechanisms, Django developers ensure the interface is seamless and efficient, making user interaction with your web application a breeze.
Understanding Django Forms
At their core, Django Forms are a simple yet powerful way to define forms, which are collections of fields where users can enter data. With Django Forms, you can validate the incoming data, display the form as HTML, and use the form’s data in your views and models. Django Forms are an integral part of Django’s Model-View-Template (MVT) architecture, providing an easy-to-use bridge between the user-facing views and the data-storing models.
Creating a Django Form
Creating a Django form is as simple as defining a Python class that inherits from `forms.Form` or `forms.ModelForm`. The former is a basic form, while the latter is used for forms tied directly to models. Let’s start by creating a basic form.
```python from django import forms class ContactForm(forms.Form): name = forms.CharField(max_length=100) email = forms.EmailField() message = forms.CharField(widget=forms.Textarea)
In the above example, we define a `ContactForm` with three fields: name, email, and message. Django provides a variety of field types, like `CharField`, `EmailField`, and many others, which help ensure that the data entered by the user is of the right type and format. The `widget` parameter allows us to control how the field is rendered in HTML.
Rendering Django Forms in Templates
Once a form is defined, you can use it in your views and templates. Here’s an example of how you might use `ContactForm` in a view:
```python from django.shortcuts import render from .forms import ContactForm def contact_view(request): if request.method == 'POST': form = ContactForm(request.POST) if form.is_valid(): # Process the form data pass else: form = ContactForm() return render(request, 'contact.html', {'form': form})
In the view, we first check if the request method is ‘POST’. If it is, we initialize the form with the POST data and validate it. If the form is valid, we can process the data. If the request method is not ‘POST’, or the form is invalid, we create a new, empty form.
In your template, you can then render the form. Django forms can be rendered as table rows, list items, or paragraphs, but you can also render them manually for full control over the HTML.
```html <form method="post"> {% csrf_token %} {{ form.as_p }} <button type="submit">Send</button> </form>
Using ModelForm
While `forms.Form` is powerful, `forms.ModelForm` provides additional functionality for forms tied directly to models. `ModelForm` automatically generates a form field for each model field specified, and it includes methods for saving the form data to the model.
Here’s an example of a `ModelForm`:
```python from django import forms from .models import ContactMessage class ContactMessageForm(forms.ModelForm): class Meta: model = ContactMessage fields = ['name', 'email', 'message']
In the `Meta` inner class, we define the model the form is tied to and
the fields we want to include. You can then use `ContactMessageForm` in your view just like `ContactForm`. The `is_valid()` method will validate both the form fields and the model fields, and the `save()` method will save the form data to a new `ContactMessage` instance.
Customizing Form Appearance
Django’s form rendering functions are convenient, but they don’t offer a lot of control over the form’s appearance. For more control, you can render the form fields manually.
```html <form method="post"> {% csrf_token %} <label for="{{ form.name.id_for_label }}">Your name:</label> {{ form.name }} <!-- Repeat for other fields --> <button type="submit">Send</button> </form>
Here, we’re rendering each form field individually, which gives us full control over the HTML.
Advanced Form Features
Beyond the basics, Django forms offer a vast array of advanced features that are fundamental for enhancing the user experience and functionality of your application. Features such as formsets (collections of forms), multi-part forms (useful for file uploads), and form wizards (multi-step forms) showcase the framework’s ability to handle complex scenarios. This is one of the many reasons why businesses choose to hire Django developers.
In addition, Django forms come equipped with comprehensive validation features. These range from simple field-level validation to complex form-wide validation, reinforcing the security and integrity of user input data. When you hire Django developers, they can leverage these advanced features to ensure your web application is not only highly interactive but also secure and reliable. These professionals understand how to best utilize the Django framework, ensuring you get the most out of its capabilities.
For instance, you can customize the validation of a form by overriding the form’s `clean()` method:
```python class ContactForm(forms.Form): # fields... def clean(self): cleaned_data = super().clean() email = cleaned_data.get('email') if "example.com" in email: raise forms.ValidationError("Email addresses from example.com are not accepted.")
In this example, we add a custom validation rule that raises a `ValidationError` if the email address contains “example.com”.
Conclusion
Django Forms are an incredibly powerful tool for handling user input in Django applications, offering an easy way to define, validate, and process forms. This rich feature set is one of the many reasons why businesses choose to hire Django developers. The expertise of these professionals in handling Django Forms helps to ensure the effective utilization of these tools for more complex use cases.
By properly understanding and using Django Forms, or by hiring skilled Django developers, you can make your web application not just more interactive, but also more secure and user-friendly. These professionals know how to harness the power of Django Forms, transforming them into highly efficient tools that elevate the overall functionality and experience of your web application. Hiring Django developers can thus be a strategic move towards creating robust, secure, and highly responsive web applications.
Table of Contents
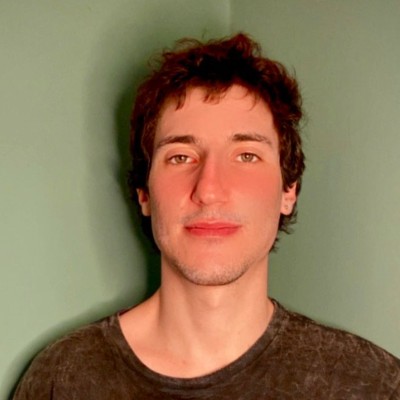
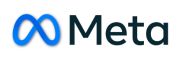