Django Unleashed: Harnessing the Full Potential of the Framework
Django, a powerful Python framework, is revered for its simplicity and robustness, making the decision to hire Django developers a prudent choice for any business. Packed with features that streamline web development, Django stands tall, personifying Python’s ethos – ‘Simple is better than complex.’ Whether you’re looking to build a complex e-commerce site or a simple blog, hiring Django developers can help you get the job done efficiently.
This blog post explores how to maximize the potential of Django, giving you a clear idea of the powerful tool your Django developers will be wielding.
An Overview of Django
Django adopts a high-level, MVC (Model-View-Controller) architectural pattern, with a “don’t repeat yourself” (DRY) principle at its core. The framework emphasizes the reusability and pluggability of components, which allows projects to be divided into standalone, reusable applications.
Exploiting Django’s Modularity
Django’s modularity, a key reason many organizations choose to hire Django developers, allows for the construction of applications as separate entities, similar to Lego blocks. Each block, expertly crafted by Django developers, performs a distinct function, and when combined, they form a fully functional, coherent system. This ability to compartmentalize and yet synchronize various application components is a testament to the power and flexibility you command when you hire Django developers.
Creating Reusable Django Apps
Consider a blog system. One Django app could handle user authentication, another could manage blog posts, other comments, and so forth. Each one is a reusable app. This way, the user authentication app could be reused in another project, without the need for rewriting code.
```python # A basic reusable app for blog posts could look like this: # models.py from django.db import models class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() # views.py from django.views import generic from .models import Post class PostListView(generic.ListView): model = Post
Using Django’s Middleware
Middleware, a series of hooks into Django’s request/response processing, is a light, low-level plugin system for globally altering Django’s input or output.
```python # Django middleware example # Adding a custom middleware to append a custom HTTP header to every response class CustomHeaderMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): response = self.get_response(request) response["Custom-Header"] = "Custom Value" return response
Unlocking Django ORM’s Potential
Django’s ORM lets developers interact with their database like you would with SQL.
Understanding QuerySets
QuerySets allow you to read the data from your database, filter it, and order it.
```python # QuerySet example from django.db.models import Q from myapp.models import Blog # Get all Blogs that contain 'django' in the title and are published today blogs = Blog.objects.filter(Q(title__contains='django') & Q(published_date=date.today()))
Using Database Relationships
Django’s ORM lets you create relationships between your models.
```python # Database relationship example from django.db import models class Blog(models.Model): name = models.CharField(max_length=100) class Entry(models.Model): blog = models.ForeignKey(Blog, on_delete=models.CASCADE) headline = models.CharField(max_length=255)
Leveraging Django’s Template Engine
Django’s template engine provides a powerful tool for dynamically outputting HTML.
Mastering Template Inheritance
Template inheritance lets you build a base template that contains all the common elements of your site.
```html <!-- Base template (base.html) --> <html> <head> <title>{% block title %}{% endblock %}</title> </head> <body> {% block content %}{% endblock %} </body> </html> <!-- Child template (child.html) --> {% extends "base.html" %} {% block title %}Home Page{% endblock %} {% block content %} <h1>Welcome to our website!</h1> <p>This is the home page.</p> {% endblock %}
Utilizing Custom Template Tags and Filters
Custom template tags and filters allow you to perform custom tasks.
``python # Custom filter to add 'rd', 'th', 'st' to day of the month from django import template register = template.Library() @register.filter def add_suffix(value): if 4 <= value <= 20 or 24 <= value <= 30: return str(value) + "th" else: return str(value) + ["st", "nd", "rd"][value % 10 - 1]
Securing Your Django Applications
Django’s security system helps protect against common attack vectors.
Using Django’s CSRF Protection
Django includes easy-to-use protection against most types of CSRF attacks.
```html <!-- Always include the CSRF token in your forms --> <form method="post"> {% csrf_token %} <!-- Form inputs go here --> </form>
Leveraging Django’s User Authentication System
Django’s robust authentication system handles user accounts, groups, permissions, and cookie-based user sessions.
```python # Using Django's built-in login view from django.contrib.auth import views as auth_views # In your urls.py path('login/', auth_views.LoginView.as_view(), name='login'),
Conclusion
Django’s ‘included batteries’ approach ensures that everything you need for comprehensive web development is at your fingertips. The key lies in understanding and utilizing these features effectively. By exploiting Django’s modularity, unlocking the ORM’s potential, leveraging the template engine, and securing your applications, you can harness Django’s full potential. This leads to clean, efficient, and maintainable code – a compelling reason for many businesses to hire Django developers. The decision to hire Django developers not only affords you access to these comprehensive tools and features but also brings onboard the necessary expertise to apply them to their maximum effect.
Table of Contents
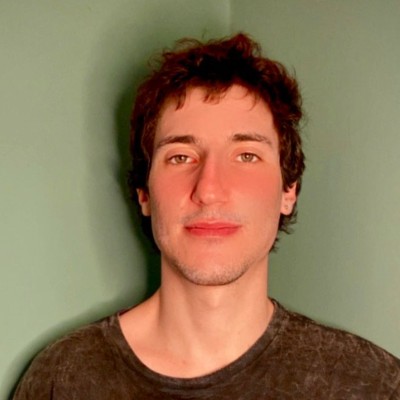
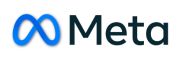