How to use Django with GraphQL for API development?
Integrating Django with GraphQL is a powerful way to develop APIs that allow clients to request exactly the data they need, reducing over-fetching and under-fetching of data. Here’s a step-by-step guide on how to use Django with GraphQL for API development:
- Install Required Packages:
Start by installing the necessary packages. You’ll need `graphene-django` for integrating GraphQL into Django. You can also use `django-graphql-jwt` for JWT-based authentication
```bash pip install graphene-django django-graphql-jwt ```
- Create a GraphQL Schema:
Define your GraphQL schema using Graphene-Django. Create a Python file, for example, `schema.py`, where you define your types, queries, and mutations. These types should map to your Django models.
```python import graphene from graphene_django.types import DjangoObjectType from .models import YourModel class YourModelType(DjangoObjectType): class Meta: model = YourModel class Query(graphene.ObjectType): all_your_models = graphene.List(YourModelType) def resolve_all_your_models(self, info): return YourModel.objects.all() schema = graphene.Schema(query=Query) ```
- Configure URLs:
In your Django project’s `urls.py`, create a URL pattern to handle GraphQL queries. This will route incoming GraphQL requests to the schema you defined.
```python from django.urls import path from graphene_django.views import GraphQLView urlpatterns = [ path('graphql/', GraphQLView.as_view(graphiql=True, schema=schema)), ] ```
- Testing GraphQL API:
You can now run your Django project and access the GraphQL interface at `/graphql/`. Use this interface to test your queries, mutations, and retrieve data from your database.
- Authentication (Optional):
If you want to implement authentication, you can use packages like `django-graphql-jwt` to add JWT-based authentication to your GraphQL API.
- Optimizing Queries:
GraphQL allows clients to specify exactly which fields they need. It’s essential to optimize your queries to prevent over-fetching. Tools like DataLoader and query analysis can help with this.
- Pagination and Authorization:
Implement pagination and authorization logic as per your application’s requirements. You can use Django’s built-in features for pagination and create custom logic for authorization.
- Documentation:
Consider using tools like Graphene-Django’s built-in documentation or third-party libraries to generate documentation for your GraphQL API. Documentation is crucial for helping other developers understand how to use your API effectively.
By following these steps, you can integrate GraphQL into your Django project and create a flexible and efficient API that allows clients to request data in a more granular and efficient manner.
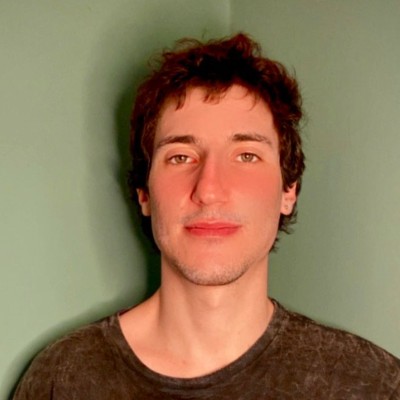
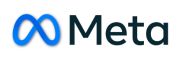