How to handle versioning in a Django REST framework API?
Handling API versioning in a Django REST framework (DRF) application is essential to ensure backward compatibility and allow changes and improvements to the API without breaking existing client applications. There are several approaches to API versioning in DRF:
- URL Path Versioning:
One common approach is to include the API version in the URL path. For example:
``` /api/v1/resource/ /api/v2/resource/ ```
To implement this, you can create different views and serializers for each version of the API and route requests accordingly in your URL configuration.
- Accept Header Versioning:
Another method is to specify the version in the HTTP Accept header of the request. Clients can request a specific version like this:
``` GET /resource/ HTTP/1.1 Host: example.com Accept: application/vnd.myapi.v1+json ```
In your DRF views, you can examine the Accept header and respond accordingly by using custom serializers or view classes for each version.
- Query Parameter Versioning:
API versions can also be specified using query parameters. For example:
``` /api/resource/?version=1 ```
In your views, you can inspect the `version` query parameter and route requests to the appropriate version.
- Header Versioning:
Some APIs use custom headers to indicate the version. For instance:
``` GET /resource/ HTTP/1.1 Host: example.com X-API-Version: 1 ```
You can access the custom header in your views and apply version-specific logic.
- Namespace Versioning:
You can organize your views and serializers into separate namespaces or packages for each API version. This method keeps version-specific code isolated.
- Deprecation and Sunsetting:
When introducing a new version, it’s essential to communicate deprecation and sunsetting plans for the older version. Notify developers and provide migration guides to facilitate a smooth transition.
Choose the versioning method that best suits your project’s requirements and maintain consistency throughout your API. Additionally, document your API versioning strategy thoroughly to help developers understand how to interact with different versions of your API effectively.
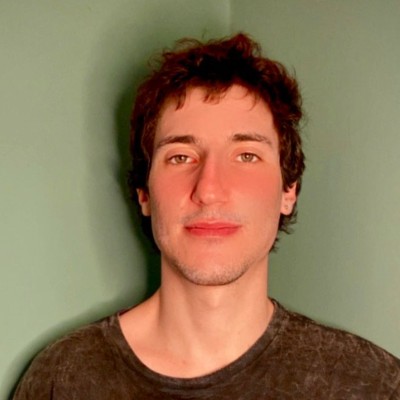
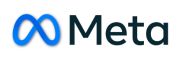