Django & Pillow: Crafting Stunning Web Images Made Easy
Image processing is a prominent feature of many modern web applications. Whether it’s photo editing platforms, e-commerce websites, or social media, there’s a high chance some form of image processing is taking place in the background. Django, one of the most popular web frameworks in Python, is no exception. Coupled with Python’s powerful imaging library, Pillow, Django can easily cater to the image processing needs of any application. Let’s explore how.
Table of Contents
1. Setting up your Django Environment
Before diving in, ensure that you’ve got Django and Pillow installed. If not, they can be installed via pip:
```bash pip install django pillow ```
2. Uploading Images
The first step to image manipulation is, of course, to get an image. Django provides an `ImageField` which is tailor-made for this.
models.py
```python from django.db import models class Image(models.Model): name = models.CharField(max_length=255) image = models.ImageField(upload_to='images/') ```
Here, `upload_to` defines the sub-directory within your `MEDIA_ROOT` where uploaded images will be stored.
Remember to adjust your settings:
settings.py
```python MEDIA_URL = '/media/' MEDIA_ROOT = os.path.join(BASE_DIR, 'media/') ```
And don’t forget to add and configure the media root in `urls.py`.
3. Image Manipulation with Pillow
Once the image is uploaded, you can begin with the manipulation. Python’s Pillow library offers a myriad of operations.
3.1. Resizing an Image
To maintain consistent image sizes across the site:
```python from PIL import Image as PilImage def resize_image(image_path, base_width): img = PilImage.open(image_path) w_percent = base_width / float(img.width) h_size = int(float(img.height) * float(w_percent)) img = img.resize((base_width, h_size), PilImage.ANTIALIAS) img.save(image_path) ```
3.2. Applying Filters
Want a black and white image? Easy!
```python def apply_grayscale(image_path): img = PilImage.open(image_path) grayscale_img = img.convert("L") grayscale_img.save(image_path) ```
4. Integrate Image Processing in Django Views
Once your functions are ready, you can integrate them into your Django views.
views.py
```python from django.shortcuts import render, redirect from .models import Image from .utils import resize_image, apply_grayscale # Assuming you've saved the functions in a utils.py def upload_and_process_image(request): if request.method == 'POST': img = Image(name=request.POST['name'], image=request.FILES['image']) img.save() # Resize and apply grayscale image_path = img.image.path resize_image(image_path, 500) # resizing to 500 width apply_grayscale(image_path) return redirect('image_view', img.id) return render(request, 'upload.html') ```
In this example, as soon as the image is uploaded, it’s resized and turned grayscale.
5. Advanced Manipulations
Pillow doesn’t stop at basic operations; you can also:
5.1. Rotate Images
```python def rotate_image(image_path, angle): img = PilImage.open(image_path) img = img.rotate(angle) img.save(image_path) ```
5.2. Crop Images
```python def crop_image(image_path, coordinates): img = PilImage.open(image_path) cropped_img = img.crop(coordinates) # coordinates as (left, upper, right, lower) cropped_img.save(image_path) ```
Conclusion
Django and Pillow together form a powerful duo for web-based image processing. With Django managing the web infrastructure and Pillow providing extensive image operations, the possibilities are vast. The examples provided here are just the tip of the iceberg. With more exploration, you can uncover advanced manipulations like drawing, color adjustments, and even format conversions. Dive in and unlock the world of image processing for your Django apps!
Table of Contents
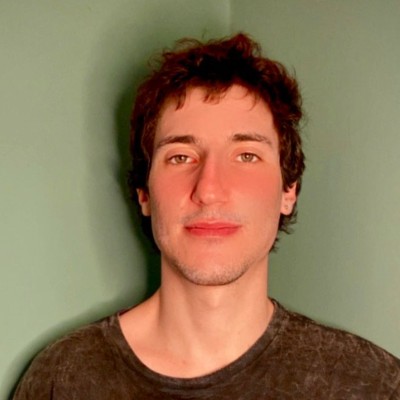
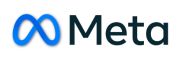