Django Q & A
How to implement pagination in Django?
Implementing pagination in Django is essential when dealing with a large set of data that needs to be displayed across multiple pages to enhance user experience and reduce loading times. Django provides built-in support for pagination through the `Paginator` class and template tags. Here’s how to implement pagination in Django:
- Import Necessary Modules: In your view function, import the necessary modules:
```python from django.core.paginator import Paginator from django.shortcuts import render ```
- Paginate Queryset: First, retrieve the data you want to paginate as a queryset. For example:
```python items = YourModel.objects.all() ```
- Initialize Paginator: Create an instance of the `Paginator` class, passing in the queryset and the number of items to display per page:
```python paginator = Paginator(items, per_page) ```
- Get Current Page Number: Determine the current page number from the request’s GET parameters:
```python page_number = request.GET.get('page') ```
- Get Page Object: Retrieve the specific page of data using the `get_page()` method:
```python page = paginator.get_page(page_number) ```
- Pass Page Object to Template: Finally, pass the `page` object to your template context:
```python return render(request, 'your_template.html', {'page': page}) ```
- Template Integration: In your template, use the `{% for %}` template tag to loop through the items on the current page. Additionally, you can use the provided template tags like `{% if page.has_previous %}` and `{% if page.has_next %}` to generate navigation links for previous and next pages.
```html {% for item in page %} <!-- Render item here --> {% endfor %} <div class="pagination"> <span class="step-links"> {% if page.has_previous %} <a href="?page=1">« first</a> <a href="?page={{ page.previous_page_number }}">previous</a> {% endif %} <span class="current-page">{{ page.number }}</span> {% if page.has_next %} <a href="?page={{ page.next_page_number }}">next</a> <a href="?page={{ page.paginator.num_pages }}">last »</a> {% endif %} </span> </div> ```
By following these steps, you can efficiently implement pagination in Django, allowing users to navigate through large datasets with ease. Pagination enhances the user experience by breaking down content into manageable chunks and reducing page load times.
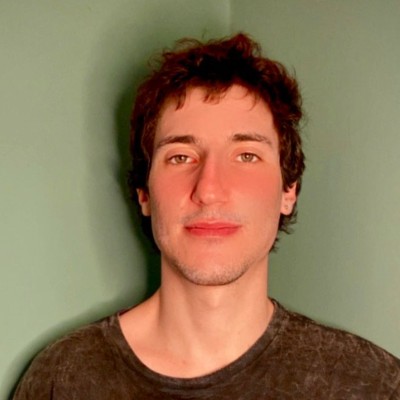
Previously at
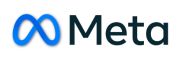
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.