Django and Angular: An Unbeatable Combination for Web App Development
In today’s modern web development era, designing web applications that provide interactive, dynamic, and efficient user experiences is crucial. As the demand grows, businesses increasingly seek to hire Django developers who can proficiently handle back-end technologies. Django, paired with powerful front-end frameworks like Angular, has become a popular approach in the industry. This article delves into the concept of integrating Django with Angular, providing practical examples to help aspiring and professional Django developers navigate this integration.
Table of Contents
1. Introduction to Django and Angular
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Built by experienced developers, it handles much of the hassle of web development, enabling developers to focus on writing their app without needing to reinvent the wheel.
On the other hand, Angular is a robust front-end JavaScript framework developed by Google. It simplifies the development process and fosters effective web applications by offering a fantastic suite of tools and features such as two-way data binding, modular architecture, reactive forms, and much more.
2. Why Combine Django and Angular?
While Django offers an excellent administrative interface, its templating system may not be enough to build highly interactive web apps. Angular complements Django by empowering the creation of single-page applications (SPAs), offering dynamic updates, and providing a rich user experience.
The synergy of Django’s powerful back-end capabilities and Angular’s seamless front-end solutions results in the development of modern, scalable, and robust web applications.
3. Setting Up the Development Environment
Before diving into the integration, ensure that you have the following installed on your system:
– Python 3.6 or higher
– Django
– Node.js and npm
– Angular CLI
You can install Django using pip: `pip install django`.
For Angular, first, install Node.js and npm. You can then install the Angular CLI globally using npm: `npm install -g @angular/cli`.
4. Creating the Django Backend
Let’s begin by creating a new Django project.
``` django-admin startproject django_angular_project cd django_angular_project ```
Next, create an app within the project.
``` python manage.py startapp django_angular_app ```
After creating your app, add it to the installed apps in `settings.py`:
```python INSTALLED_APPS = [ ... 'django_angular_app', ... ] ```
Create a model in `models.py`:
```python from django.db import models class Task(models.Model): title = models.CharField(max_length=200) description = models.TextField() def __str__(self): return self.title ```
After creating your model, run the migrations.
``` python manage.py makemigrations python manage.py migrate ```
Next, we’ll create an API using Django Rest Framework (DRF) to serve our data to the Angular app. Install DRF: `pip install djangorestframework`.
Add `rest_framework` to the `INSTALLED_APPS` in `settings.py`:
```python INSTALLED_APPS = [ ... 'rest_framework', ... ] ```
Create a serializer in `serializers.py`:
```python from rest_framework import serializers from .models import Task class TaskSerializer(serializers.ModelSerializer): class Meta: model = Task fields = ('id', 'title', 'description') ```
Next, create a viewset in `views.py`:
```python from rest_framework import viewsets from .models import Task from .serializers import TaskSerializer class TaskViewSet(viewsets.ModelViewSet): queryset = Task.objects.all() serializer_class = TaskSerializer ```
In `urls.py`, set up the URL routing for the API:
```python from django.urls import path, include from rest_framework.routers import DefaultRouter from .views import TaskViewSet router = DefaultRouter() router.register(r'tasks', TaskViewSet) urlpatterns = [ path('', include(router.urls)), ] ```
Now, you can run the Django server: `python manage.py runserver`. You should be able to access the API at `http://127.0.0.1:8000/`.
5. Creating the Angular Frontend
Let’s create a new Angular application:
``` ng new angular_frontend cd angular_frontend ```
You can start the Angular server with `ng serve`. By default, the Angular server runs on port 4200.
Let’s create a service to communicate with the Django backend:
``` ng generate service task ```
This will create `task.service.ts`. In this file, define methods to interact with the API:
```typescript import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class TaskService { private baseUrl = 'http://127.0.0.1:8000/'; constructor(private http: HttpClient) { } getTaskList(): Observable<any> { return this.http.get(`${this.baseUrl}tasks/`); } createTask(task: Object): Observable<Object> { return this.http.post(`${this.baseUrl}tasks/`, task); } // Add other methods for update, delete etc. } ```
Next, generate a component to handle tasks:
``` ng generate component task-list ```
In `task-list.component.ts`, inject the `TaskService` and call the API:
```typescript import { Component, OnInit } from '@angular/core'; import { TaskService } from '../task.service'; @Component({ selector: 'app-task-list', templateUrl: './task-list.component.html', styleUrls: ['./task-list.component.css'] }) export class TaskListComponent implements OnInit { tasks: any; constructor(private taskService: TaskService) { } ngOnInit() { this.taskService.getTaskList().subscribe(data => { this.tasks = data; }); } } ```
In `task-list.component.html`, you can loop through the tasks and display them:
```html <div *ngFor="let task of tasks"> <h2>{{task.title}}</h2> <p>{{task.description}}</p> </div> ```
Similarly, you can create components for adding, updating, and deleting tasks.
Conclusion
By integrating Django and Angular, you can leverage the best of both worlds – Django’s powerful back-end capabilities, including handling databases and rendering server-side pages, and Angular’s dynamic and interactive front-end features. This is why companies are increasingly looking to hire Django developers who are skilled in such integrations. While this tutorial provided a simple example of integrating Django with Angular, with more complexity, customization, and an experienced Django developer on board, you can create high-performance, feature-rich web applications. Happy coding!
Table of Contents
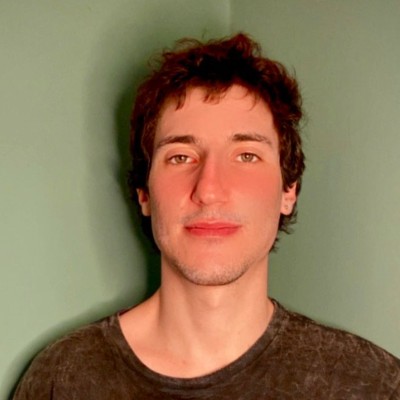
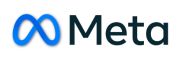