What is logging system, and how to configure it?
Django’s logging system is a powerful tool that allows developers to track and record various events, errors, and information within a Django application. Properly configured logging is essential for debugging, monitoring, and maintaining the health of your application. Here’s an overview of Django’s logging system and how to configure it:
- Logging Configuration:
Django’s logging settings are typically defined in the `settings.py` file of your project. You can customize the logging behavior by configuring various aspects, such as loggers, handlers, formatters, and log levels.
```python LOGGING = { 'version': 1, 'disable_existing_loggers': False, 'handlers': { 'file': { 'level': 'DEBUG', 'class': 'logging.FileHandler', 'filename': 'django.log', }, }, 'loggers': { 'django': { 'handlers': ['file'], 'level': 'DEBUG', 'propagate': True, }, }, } ```
In this example, we define a simple file-based logging configuration. You can customize it to use different log levels (e.g., DEBUG, INFO, WARNING, ERROR, CRITICAL), specify different handlers (e.g., file, console, email), and format log messages according to your requirements.
- Log Messages:
You can generate log messages from various parts of your Django application, including views, models, middleware, and custom modules. To log a message, use Python’s built-in `logging` module, which Django integrates with. For example:
```python import logging logger = logging.getLogger(__name__) def my_function(): # Your code here logger.debug('Debug message') logger.info('Informational message') logger.warning('Warning message') logger.error('Error message') logger.critical('Critical message') ```
- Log Routing:
Django’s logging system allows you to route log messages to different handlers and control their verbosity based on log levels. You can also specify whether log messages should propagate to parent loggers.
- Third-Party Libraries:
Many third-party packages and services integrate seamlessly with Django’s logging system. For example, you can configure log handlers to send log entries to external services like Elasticsearch, Logstash, or Sentry for advanced monitoring and debugging.
- Best Practices:
It’s crucial to follow logging best practices, including proper log level selection, meaningful log messages, and careful handling of sensitive information.
Django’s logging system is a valuable tool for diagnosing issues, monitoring application health, and improving code quality. By configuring it to suit your application’s needs and using it consistently, you can streamline the debugging process and gain insights into your application’s behavior.
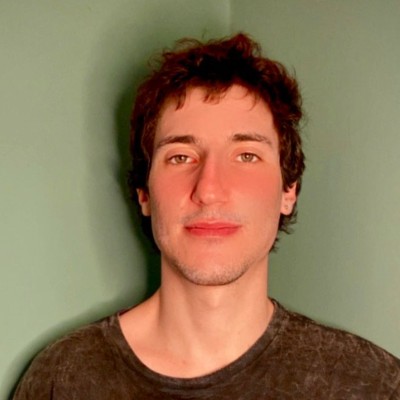
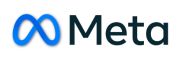