Django Coding Essentials: Best Practices for Maintainable Code
Django, a high-level Python Web framework, encourages rapid development and clean, pragmatic design. Known for its many strengths, including its adherence to the DRY (Don’t Repeat Yourself) principle and its focus on writing maintainable code, it’s a popular choice for organizations looking to hire Django developers. However, while Django promotes these practices, it’s still up to the developer to ensure that their code is clean, maintainable, and efficient.
In this blog post, we will explore some best practices that can help you, or the Django developers you hire, write more elegant, efficient, and maintainable Django code. These principles are applicable no matter what kind of project you’re working on – a small personal project or a large commercial one. Let’s dive right in.
1. Leverage Django’s Built-in Features
One of Django’s biggest strengths is its wide array of built-in features that can simplify development and reduce the amount of code you need to write.
Take Django’s ORM, for example. It allows you to interact with your database, like you would with SQL. In other words, it’s a way to create, retrieve, update, and delete records in your database using Python.
Let’s say you have a model called `BlogPost`, and you want to retrieve all the blog posts that are published. Instead of writing raw SQL queries, you can use Django’s ORM to achieve the same result:
```python # Bad practice BlogPost.objects.raw('SELECT * FROM myapp_blogpost WHERE status = "published"') # Good practice BlogPost.objects.filter(status="published") ```
In the above example, Django’s ORM provides a more Pythonic, readable way of performing database operations. It also helps prevent common security issues, such as SQL injection attacks.
2. Keep Your Models Skinny
Keeping your models skinny is a crucial aspect of maintaining clean, maintainable code in Django. A common anti-pattern is to stuff too much logic into the model or view layer. This makes your code harder to understand, test, and maintain.
Instead, business logic should be pushed down into service layers or utility functions.
```python # Bad practice class BlogPost(models.Model): ... def publish(self): self.status = "published" self.publish_date = timezone.now() self.save() send_email_to_subscribers(self) # Good practice class BlogPost(models.Model): ... def publish(self): self.status = "published" self.publish_date = timezone.now() self.save() def send_email_for_new_post(post): send_email_to_subscribers(post) # Now when you want to publish a post, you'd do: post = BlogPost.objects.get(id=1) post.publish() send_email_for_new_post(post) ```
In the “good practice” example, the `BlogPost` model doesn’t need to know anything about how to email subscribers. This approach makes the code more modular and easier to test and maintain.
3. Utilize Django’s Form and ModelForm
Django forms are an excellent tool for handling form data and validating it before saving it into the database. They can also save you from writing repetitive HTML form code.
A `ModelForm` is a helper class that lets you create a `Form` class from a Django model. Here’s how you can leverage it:
```python # models.py class BlogPost(models.Model): title = models.CharField(max_length=100) content = models.TextField() # forms.py from django import forms from .models import BlogPost class BlogPostForm(forms.ModelForm): class Meta: model = BlogPost fields = ['title', 'content'] # views.py from django.shortcuts import render from .forms import BlogPostForm def create_blogpost(request): if request.method == 'POST': form = BlogPostForm(request.POST) if form.is_valid(): form.save() return redirect('blogpost_list') else: form = BlogPostForm() return render(request, 'create_blogpost.html', {'form': form}) ```
In this example, `BlogPostForm` automatically generates form fields from the `BlogPost` model. This saves you from having to manually define the form fields yourself and ensure they match up with your model fields. Django’s forms also handle form validation, so you can be sure that the data you’re getting is clean and safe.
4. Employ Class-Based Views
Django’s class-based views provide a more organized and OOP way to handle HTTP requests. By using class-based views, you can organize your views according to the kind of action they perform.
Consider the following example:
```python # Function-based view from django.shortcuts import render, get_object_or_404 from .models import BlogPost def blogpost_detail(request, pk): blogpost = get_object_or_404(BlogPost, pk=pk) return render(request, 'blogpost_detail.html', {'blogpost': blogpost}) # Class-based view from django.views import View from django.shortcuts import render, get_object_or_404 from .models import BlogPost class BlogPostDetailView(View): def get(self, request, *args, **kwargs): blogpost = get_object_or_404(BlogPost, pk=kwargs['pk']) return render(request, 'blogpost_detail.html', {'blogpost': blogpost}) ```
In the class-based view, HTTP methods (GET, POST, etc.) are explicitly separated, making it easier to handle different request types. Class-based views also come with a variety of useful built-in generic views, like `ListView`, `DetailView`, `CreateView`, `UpdateView`, and `DeleteView`.
5. Proper Use of Middleware
Middleware is a series of hooks into Django’s request/response processing. It’s a lightweight, low-level plugin system for globally altering Django’s input or output.
Consider a scenario where you need to add a custom header ‘X-Frame-Options’ to every response to prevent clickjacking. You can easily handle this using Django middleware:
```python # myapp/middleware.py class XFrameOptionsMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): response = self.get_response(request) response['X-Frame-Options'] = 'DENY' return response # settings.py MIDDLEWARE = [ ... 'myapp.middleware.XFrameOptionsMiddleware', ... ] ```
Middleware lets you process requests and responses globally before they reach the view or after they leave the view, saving you the effort of writing the same piece of code in multiple views.
Conclusion
Django provides a robust foundation for building web applications. This is why many companies opt to hire Django developers who can fully utilize these features to their maximum potential, ensuring the codebase remains maintainable and efficient. By keeping in mind the principles we’ve discussed here, whether you’re a solo coder or part of a team hiring Django developers, your Django code will be clean, efficient, and, most importantly, easy to understand for anyone who might work on the project in the future. The key to success is in the mastery of these best practices. Happy coding!
Table of Contents
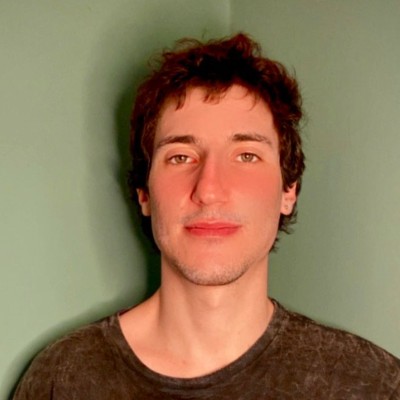
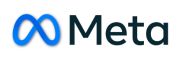