Boost Your Web Application’s Performance with Django and Microservices
In the realm of contemporary software development, two major trends have quickly gained prominence due to their abilities to simplify and accelerate processes: Django and Microservices. Django, a high-level Python web framework, facilitates rapid development and practical, clean design. Microservices, on the other hand, is an architectural style that organizes an application as a collective of services. A smart move would be to hire Django developers who can effectively leverage these two powerful tools. This post explores how these two entities can be combined to construct modular and scalable architectures, enriched with practical examples. With the right team of Django developers on board, you can harness the full potential of these technologies and drive your project to success.
Table of Contents
1. An Overview of Django
Django is a web framework for perfectionists (with deadlines). It follows the Model-View-Controller (MVC) design pattern and provides a foundation for building scalable and maintainable applications. Django includes all the necessary components like an ORM (Object Relational Mapping), authentication, and routing, allowing developers to focus on writing the business logic and interface rather than boilerplate code.
2. An Overview of Microservices
Microservices, also known as the microservice architecture, is an architectural style that structures an application as a collection of services that are highly maintainable and testable, loosely coupled, independently deployable, and organized around business capabilities. The microservice architecture enables the continuous delivery and deployment of large, complex applications.
Now that we have a basic understanding of Django and Microservices, let’s see how they can be combined to create robust architectures.
3. Django and Microservices
Despite its monolithic nature, Django is perfectly capable of working in a microservice architecture. Here’s how we can achieve it:
Example : User Authentication Microservice
Consider a simple application with various functionalities, such as user registration, login, and article management.
Let’s create a Django project called “UserService” which only manages user authentication.
3.1 Setting Up the Project
Create a new Django project:
```shell django-admin startproject UserService ```
Navigate to the newly created project:
```shell cd UserService ```
Create a new app:
```shell python manage.py startapp authentication ```
3.2. Creating Models
In `authentication/models.py`, we define a User model:
```python from django.db import models from django.contrib.auth.models import AbstractUser class User(AbstractUser): email = models.EmailField(unique=True) ```
3.3. Creating Views
In `authentication/views.py`, we define views for registration and login:
```python from django.contrib.auth import get_user_model from django.contrib.auth import authenticate from django.views.decorators.csrf import csrf_exempt from rest_framework.authtoken.models import Token from rest_framework.response import Response from rest_framework.views import APIView User = get_user_model() class RegistrationAPIView(APIView): ... class LoginAPIView(APIView): ... ```
3.4. Deploying Microservice
This Django project is now a self-contained microservice for user authentication. It can be deployed independently from other microservices, ensuring that changes in other areas of the application will not affect it.
Example: Article Management Microservice
Let’s create another Django project called “ArticleService”, which is solely responsible for article management.
The creation and structure of this microservice will be similar to the “UserService” example. Here, we’ll define an Article model and corresponding views for adding, updating, and removing articles.
The crucial point to note here is the independence of these microservices. They can communicate with each other when needed, but they are not tightly coupled. Changes in one microservice will not directly impact the others, enhancing the flexibility and scalability of our overall architecture.
4. Communication between Microservices
Although our microservices are independent, they still need to communicate with each other for certain operations. For instance, before creating an article, the ArticleService needs to ensure the user is authenticated.
This communication can be achieved via APIs or message brokers. Using APIs, one microservice can send a request to another and get a response. With message brokers, microservices can publish and subscribe to messages.
Let’s create an example with API communication.
When creating an article, the ArticleService sends a request to the UserService’s authentication API. If the user is authenticated, ArticleService proceeds with the creation of the article; otherwise, it responds with an error.
Conclusion
Looking to revolutionize your web application? Django and microservices are your answers. This dynamic duo offers a comprehensive solution for modern application development, fostering the creation of modular architectures. These components can be independently developed, deployed, and scaled, enhancing the flexibility and scalability of your applications.
Hiring Django developers can help you leverage the potent tool of this traditionally monolithic framework in a microservice architecture. As our exploration has shown, it’s not only feasible but also advantageous. Django’s rapid development capabilities, married with the versatility of microservices, are a game-changer for building adaptable, maintainable applications. So, don’t wait. Hire Django developers today and kickstart your journey towards scalable and robust web development.
Table of Contents
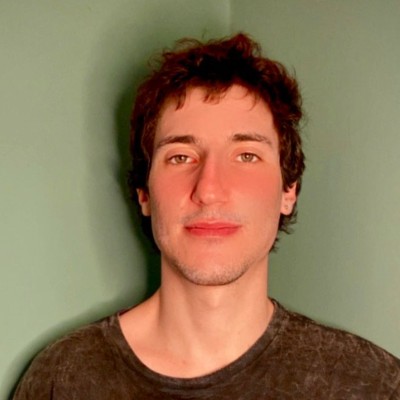
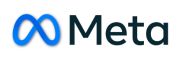