A Developer’s Guide to Securing Online Payments in Django with Stripe and PayPal
In the digital era, seamless online transactions have become an integral part of a multitude of applications. As the modern internet economy continues to grow, so does the need for secure, efficient, and reliable online payment systems. In such scenarios, the decision to hire Django developers can significantly enhance your application’s performance and security.
Table of Contents
In this blog post, we’ll examine how to integrate secure payment systems into a Django web application. Django is a high-level Python web framework that fosters rapid development and pragmatic design, making it a prime choice for businesses looking to hire Django developers. These professionals are adept at creating scalable, secure, and maintainable web applications, further solidifying Django’s reputation in the web development landscape.
1. What is a Payment Gateway?
A payment gateway is a service that sends credit card information from a website to the credit card payment networks for processing and returns transaction details and responses from the payment networks back to the website. In essence, payment gateways facilitate communication within banks.
Payment gateways help ensure that sensitive information, such as credit card numbers, entered into a virtual terminal or on an e-commerce website, is encrypted and secure, minimizing the likelihood of fraud.
2. Payment Gateway Options
There are numerous payment gateways available, each offering different features, pricing models, and regional availability. Some popular payment gateways include Stripe, PayPal, Square, and Braintree, among others. When choosing a gateway, factors such as transaction fees, payment methods, user experience, and the ease of integration with your web application should be considered.
In this blog post, we will focus on integrating Stripe and PayPal into a Django application.
3. Setting up Django for Payments
Before integrating a payment gateway, we need to have our Django application ready. Ensure that you have installed Django and created an application. If not, Django’s documentation offers a thorough guide on how to get started.
Let’s assume we are building a simple e-commerce app that has a shopping cart. We would already have models representing our `Products` and `Orders`.
4. Stripe Integration
Stripe is a well-known payment gateway that provides APIs for processing payments. It supports multiple payment types, including cards, bank transfers, and Bitcoin.
4.1 Setting Up Stripe Account
First, create an account at Stripe’s website and retrieve the API keys from the dashboard. The two keys you will need are `Publishable Key` and `Secret Key`.
4.2 Installing Stripe Python Library
To work with Stripe in our Django project, install Stripe’s Python library:
```bash pip install stripe ```
4.3 Configuring Stripe in Django
Create a new file named `stripe_config.py` in your main Django app directory. In this file, add the following lines:
```python import stripe stripe.api_key = 'your-secret-key' ```
Now, replace `’your-secret-key’` with your actual Stripe Secret Key.
4.4 Adding Stripe to Your Django Models
In the Order model, we add a `stripe_id` field which we’ll use to store the unique identifier that Stripe returns after a successful payment.
```python class Order(models.Model): # existing fields... stripe_id = models.CharField(max_length=255, blank=True, null=True) ```
4.5 Creating a Stripe Charge
Assuming we have a checkout form where users fill in their payment information, on form submission, we’ll send this information to Stripe to create a charge. We would use Stripe’s `stripe.Charge.create` method. Below is an example of how we can achieve this:
```python import stripe from .models import Order def create_charge(request): if request.method == 'POST': token = request.POST['stripeToken'] order = Order.objects.get(user=request.user, ordered=False) try: charge = stripe.Charge.create( amount=100, # amount is in cents currency='usd', source=token, description=f"Charge for {request.user.username}" ) order.ordered = True order.stripe_id = charge['id'] order.save() return redirect('success_page') except stripe.error.CardError as e: # handle card error pass ```
5. PayPal Integration
PayPal is another popular payment gateway. It’s globally recognized and trusted by millions of businesses and individuals for processing payments.
5.1 Setting Up PayPal Account
Firstly, you need to create a PayPal business account and get the API credentials (Client ID and Secret) from the developer dashboard.
5.2 Installing PayPal Python SDK
You can install the PayPal Python SDK with the following command:
```bash pip install paypalrestsdk ```
5.3 Configuring PayPal in Django
Similar to Stripe, we’ll set up a configuration file, `paypal_config.py`, and add the following:
```python import paypalrestsdk paypalrestsdk.configure({ "mode": "sandbox", # sandbox or live "client_id": "YOUR_CLIENT_ID", "client_secret": "YOUR_CLIENT_SECRET" }) ```
Replace `”YOUR_CLIENT_ID”` and `“YOUR_CLIENT_SECRET”` with your actual PayPal client ID and secret.
5.4 Creating a PayPal Payment
PayPal SDK provides a `Payment` object which we can use to create a payment. Here is an example of how to create a payment using PayPal Python SDK:
```python import paypalrestsdk from .models import Order def create_payment(request): if request.method == 'POST': order = Order.objects.get(user=request.user, ordered=False) payment = paypalrestsdk.Payment({ "intent": "sale", "payer": { "payment_method": "paypal" }, "redirect_urls": { "return_url": "http://localhost:8000/payment/execute", "cancel_url": "http://localhost:8000/" }, "transactions": [{ "item_list": { "items": [{ "name": "item", "sku": "item", "price": '10.00', "currency": "USD", "quantity": 1 }]}, "amount": { "total": '10.00', "currency": "USD" }, "description": f"Payment for {request.user.username}" }]}) if payment.create(): order.paypal_id = payment.id order.save() return redirect(payment.links[1].href) # redirect the user to PayPal checkout page else: print(payment.error) ```
Conclusion
Integrating payment gateways in a Django application might seem daunting initially, but with the right tools or the right team of skilled Django developers, the process can be relatively straightforward. Both Stripe and PayPal offer robust, secure services for processing payments, and with the provided examples, you should be well on your way to accepting online payments in your Django application.
Remember to take security seriously when dealing with transactions. Always ensure your application is running on HTTPS and be aware of PCI DSS requirements when handling card data. If these tasks appear too complex, hiring Django developers could be the optimal solution for your project’s success. Happy coding!
Table of Contents
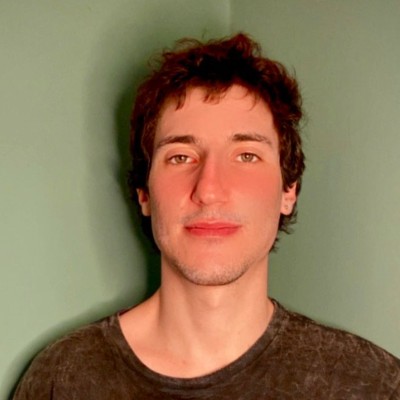
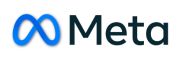