Django ORM: Simplifying Database Operations in Your Web Applications
One of the biggest perks of Django, a popular high-level Python Web framework, is its built-in Object-Relational Mapping (ORM) layer. This sophisticated tool is one of the main reasons organizations hire Django developers. An ORM is a powerful tool that facilitates the interaction between an application and a relational database by mapping Python objects to database tables. This abstraction allows developers, including those that companies hire as Django developers, to interact with their data as if they were Python objects, without having to write complex SQL queries.
In this blog post, we’ll delve into Django ORM, demonstrating its robustness, simplicity, and capabilities in facilitating database operations in your web applications, further reinforcing why businesses choose to hire Django developers.
Understanding Django ORM
At its core, Django’s ORM provides a high-level, Pythonic interface to a relational database. This powerful feature is one of the reasons why organizations frequently hire Django developers. It allows you to create, retrieve, update, and delete records in your database using Python. It also provides a query API with full support for JOIN operations, aggregations, transactions, and more.
Each table in your database corresponds to a Django model. A model is essentially a Python class, and each instance of this class represents a row in the database table. Each attribute of the model represents a field in the table. When you hire Django developers, they can leverage this functionality to streamline and optimize your web applications.
For instance, imagine you’re building a blog application. You may have a model for blog posts and another for comments. As any skilled Django developer would know, defining these in Django would look like this.
```python from django.db import models class BlogPost(models.Model): title = models.CharField(max_length=200) content = models.TextField() pub_date = models.DateTimeField('date published') class Comment(models.Model): blog_post = models.ForeignKey(BlogPost, on_delete=models.CASCADE) text = models.TextField() author = models.CharField(max_length=200)
In the above code, the `BlogPost` model has fields `title`, `content`, and `pub_date`, representing a blog post’s title, content, and publication date, respectively. The `Comment` model has a foreign key field, `blog_post`, referencing the associated `BlogPost` instance.
Django ORM’s CRUD Operations
Django’s ORM makes it straightforward to perform CRUD (Create, Read, Update, Delete) operations on the database.
Create
To create a new record in the database, you instantiate a new model and call the `save` method. For instance:
```python post = BlogPost(title="My First Post", content="Hello, Django!", pub_date=datetime.now()) post.save()
This would create a new row in the BlogPost table, with the given title, content, and publication date.
Read
Django’s ORM provides a rich API for querying the database. For instance, you can retrieve all instances of a model:
```python all_posts = BlogPost.objects.all()
Or filter them based on certain criteria:
```python recent_posts = BlogPost.objects.filter(pub_date__gte=datetime.now() - timedelta(days=7))
The above command retrieves all blog posts published in the last week.
Update
Updating a record is as easy as modifying an attribute of a model instance and calling `save`:
```python post = BlogPost.objects.get(title="My First Post") post.content = "Hello, Django! I've been updated!" post.save()
Delete
To delete a record, you call the `delete` method on a model instance:
```python post = BlogPost.objects.get(title="My First Post") post.delete()
Complex Queries and Aggregations
Django’s ORM also supports more complex queries, like joining tables, grouping records, and aggregating data. For instance, to get the number of comments for each blog post, you can use Django’s `annotate` method:
```python from django.db.models import Count posts_with_comment_count = BlogPost.objects.annotate(comment_count=Count('comment'))
Transactions
Django ORM’s capabilities extend beyond simple and complex querying. It also provides support for transactions, which are a series of database operations that are treated as a single unit. This ensures data integrity, as either all operations succeed, or if any operation fails, none are applied.
For instance, to ensure that a series of updates to a blog post and corresponding comments occur atomically, you can use Django’s `transaction` package:
``python from django.db import transaction with transaction.atomic(): post = BlogPost.objects.select_for_update().get(title="My First Post") post.content = "Hello, Django! I've been updated!" post.save() for comment in post.comment_set.all(): comment.text = "This comment was updated too!" comment.save()
In the above example, if saving any comment fails for any reason, the changes to the blog post will also be rolled back, ensuring data consistency.
Conclusion
Django’s ORM provides an incredibly powerful, yet easy-to-use interface for managing your application’s data. This unique functionality is a compelling reason why businesses choose to hire Django developers. It abstracts away many of the complexities of dealing with SQL and allows you to interact with your data as Python objects, making it more intuitive and Pythonic.
When you hire Django developers, you gain access to their skills in leveraging the ORM’s robust query API, support for transactions, and other advanced database features. With Django’s ORM, and the expertise of the Django developers you hire, database operations in your web applications can be significantly simplified and more manageable. This leaves your team free to focus on the other equally important aspects of your application.
Table of Contents
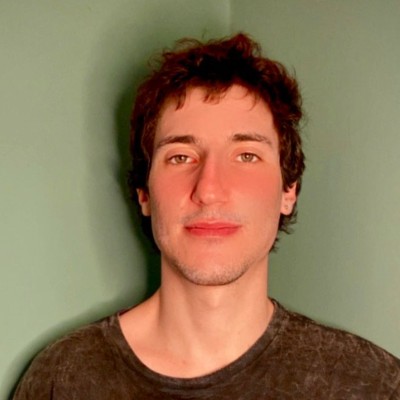
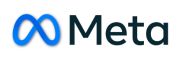