How to implement rate limiting and throttling Django REST framework API?
Implementing rate limiting and throttling in a Django REST framework (DRF) API is crucial to protect your server from abuse and ensure fair resource usage among your users. DRF provides built-in support for rate limiting and throttling, making it relatively straightforward to implement. Here’s a step-by-step guide on how to achieve this:
- Install Django Ratelimit:
Start by installing the `django_ratelimit` package using pip:
```bash pip install django_ratelimit ```
- Configure Rate Limiting:
In your DRF views, you can use the `@ratelimit` decorator to specify the rate limit for a particular view. For example, to limit a view to 100 requests per hour for each user:
```python from django_ratelimit.decorators import ratelimit @ratelimit(key='user', rate='100/hour', method='GET', block=True) def my_api_view(request): # Your view logic here ```
- Throttling Classes:
DRF provides several built-in throttling classes you can use, such as `AnonRateThrottle` (for anonymous users) and `UserRateThrottle` (for authenticated users). Configure these in your settings.py:
```python REST_FRAMEWORK = { 'DEFAULT_THROTTLE_CLASSES': [ 'rest_framework.throttling.AnonRateThrottle', 'rest_framework.throttling.UserRateThrottle', ], 'DEFAULT_THROTTLE_RATES': { 'anon': '100/hour', 'user': '1000/hour', }, } ```
- Customize Throttling:
You can create custom throttling classes by inheriting from DRF’s `SimpleRateThrottle` and implementing your logic based on your application’s requirements.
- Test and Monitor:
Thoroughly test your API with rate limiting and throttling enabled to ensure it functions as expected. Monitor server logs and usage patterns to fine-tune your rate limits and throttling settings.
By implementing rate limiting and throttling in your DRF API, you can prevent abuse, ensure fair usage, and maintain the stability and performance of your application, especially in high-traffic scenarios. Remember to strike a balance between security and usability by setting appropriate rate limits for your specific use case.
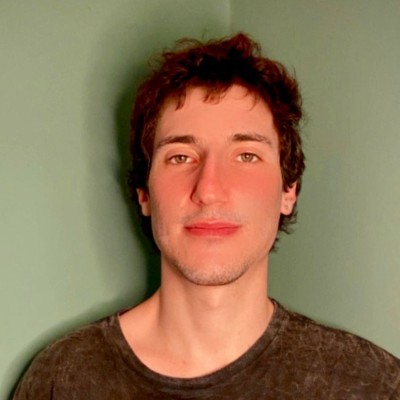
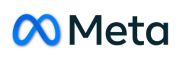