Transform Your Django App with Lightning-Fast Real-Time Analytics
Django is a powerful web framework that allows developers to build robust applications quickly. However, as the digital landscape evolves, so do user expectations. Today’s users are keen on receiving real-time feedback, updates, and information. Thus, integrating real-time analytics into Django applications is becoming more essential.
Table of Contents
This article delves deep into how you can leverage Django to track user behavior instantly and offer real-time analytics to your users. Let’s explore some compelling examples.
1. WebSocket and Django Channels
A straightforward way to get real-time capabilities in Django is by using WebSockets. They allow bi-directional communication between the server and client. With Django Channels, implementing WebSockets becomes a breeze.
Example: Real-time Page View Counter
Imagine a blog where you want to show the number of users currently viewing a particular article.
```python # models.py from django.db import models class Article(models.Model): title = models.CharField(max_length=200) content = models.TextField() viewers = models.PositiveIntegerField(default=0) ```
With Django Channels, you can increment the `viewers` field when a user opens the article and decrement it when they leave.
```python # consumers.py from channels.generic.websocket import AsyncWebsocketConsumer from .models import Article class ArticleConsumer(AsyncWebsocketConsumer): async def connect(self): self.article_id = self.scope['url_route']['kwargs']['article_id'] await self.channel_layer.group_add(self.article_id, self.channel_name) await self.accept() article = Article.objects.get(id=self.article_id) article.viewers += 1 article.save() async def disconnect(self, close_code): article = Article.objects.get(id=self.article_id) article.viewers -= 1 article.save() ```
2. Real-time Dashboards with D3.js and Django
D3.js is a JavaScript library that helps in building dynamic and interactive data visualizations. Coupled with Django, you can create mesmerizing real-time dashboards.
Example: Real-time Sales Dashboard
Imagine an e-commerce website where admins want to track sales in real-time.
First, you’d structure a model for sales:
```python # models.py class Sale(models.Model): product = models.ForeignKey(Product, on_delete=models.CASCADE) quantity = models.PositiveIntegerField() timestamp = models.DateTimeField(auto_now_add=True) ```
Then, using Django’s database hooks and D3.js, update the dashboard every time a sale happens.
```javascript // Using D3.js to update the dashboard every second d3.interval(function(){ d3.json("/api/sales/latest/").then(function(data){ // Update your dashboard with the new data. }); }, 1000); ```
3. Using Pusher with Django
Pusher is a service that provides real-time web sockets for applications. Django can work seamlessly with Pusher through libraries like `django-pusher`.
Example: Instant User Feedback on Form Submissions
Let’s consider a scenario where users submit feedback, and you wish to acknowledge them instantly.
Once the user submits feedback, use Pusher to send an immediate response.
```python # views.py from pusher import Pusher from .models import Feedback def submit_feedback(request): if request.method == "POST": feedback = Feedback(content=request.POST['content']) feedback.save() pusher = Pusher(app_id='your_id', key='your_key', secret='your_secret', cluster='your_cluster') pusher.trigger('feedback', 'submission', {'message': 'Thank you for your feedback!'})
4. Using Celery with Django for Real-time Processing
Celery is an asynchronous task queue that can process tasks in the background, making it perfect for real-time operations.
Example: Real-time Image Processing
Suppose users can upload images, and you want to process them immediately (e.g., applying filters). With Django and Celery, you can achieve this in real-time.
Once an image is uploaded, a Celery task is triggered to process the image. Post-processing, notify the user about the status.
```python # tasks.py from celery import shared_task from .models import UploadedImage @shared_task def process_image(image_id): image = UploadedImage.objects.get(id=image_id) # Your image processing logic here. image.status = "Processed" image.save() ```
Conclusion
The importance of real-time analytics and feedback in web applications cannot be understated. By incorporating real-time capabilities into Django applications, you not only enhance user experience but also get invaluable insights into user behavior instantly.
Whether you choose WebSockets with Django Channels, mesmerizing visualizations with D3.js, immediate notifications with Pusher, or background processing with Celery, Django has got you covered. Dive in and enhance your applications with real-time analytics today!
Table of Contents
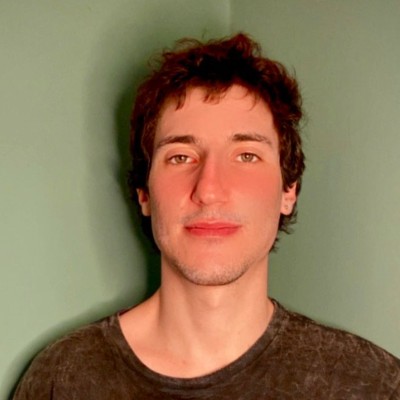
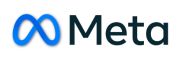