Elevate User Experiences with Django-Based Recommendation Systems
Django, the high-level Python Web framework, empowers developers to build robust and scalable web applications. One of its key strengths is its modularity and adaptability, making it suitable for a variety of use cases, from simple blogs to complex machine learning integrations. In this post, we will delve into one such integration: building recommendation systems within Django to enhance user experience.
Table of Contents
Recommendation systems play a pivotal role in the modern digital experience, driving personalized content to users on platforms such as Netflix, Amazon, and Spotify. They analyze data to predict the most suitable products, movies, songs, or articles for individual users.
1. Why Django for Recommendation Systems?
Django’s ORM (Object-Relational Mapping) system, combined with its extensibility, allows for a smooth integration of machine learning libraries and tools. This makes it relatively straightforward to build and deploy recommendation engines directly into web applications.
Example 1: Product Recommendations
Scenario: You have an e-commerce site built with Django. You want to recommend products to users based on their browsing and purchasing history.
Solution: Use collaborative filtering, a method that makes automatic predictions about the preference of a user by collecting preferences from many users.
- Collect Data: Utilize Django’s models to structure and store user interactions, such as product views, cart additions, and purchases.
- Build Recommendation Model: One of the popular Python libraries for collaborative filtering is `Surprise`. After installing it, you can build a recommendation engine:
```python from surprise import Dataset, SVD, accuracy from surprise.model_selection import train_test_split # Load dataset from Django's ORM data = Dataset.load_from_df(your_dataframe[['userId', 'productId', 'rating']], reader) # Split dataset into training and test set trainset, testset = train_test_split(data, test_size=.25) # Use the SVD algorithm algo = SVD() algo.fit(trainset) predictions = algo.test(testset) # Evaluate model accuracy.rmse(predictions) ```
- Integrate with Django: When a user logs in, retrieve recommendations:
```python recommended_products = [algo.predict(user_id, product_id) for product_id in all_products] ```
Example 2: Content Recommendations for a Blog
Scenario: You have a Django-based blog. You want to suggest articles to readers based on the articles they’ve read.
Solution: Use content-based filtering. This technique recommends items by comparing the content of the items and a user profile, with content being described in terms of several descriptors.
- Extract Features: Use `TF-IDF` to extract features from the articles. You can use Python’s `TfidfVectorizer`:
```python from sklearn.feature_extraction.text import TfidfVectorizer tfidf = TfidfVectorizer(stop_words='english') tfidf_matrix = tfidf.fit_transform(Article.objects.values_list('content', flat=True)) ```
- Compute Similarity Scores: Use cosine similarity to find similarity between articles:
```python from sklearn.metrics.pairwise import linear_kernel cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix) ```
- Make Recommendations: For an article that a user is currently reading, find the most similar ones:
```python def get_recommendations(title, cosine_sim=cosine_sim): idx = article_titles.index(title) sim_scores = list(enumerate(cosine_sim[idx])) sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True) article_indices = [i[0] for i in sim_scores[1:6]] # Get top 5 return Article.objects.filter(id__in=article_indices) ```
2. Advantages of Building Recommendation Systems in Django
- Flexibility: Django’s adaptability allows for easy integration of various recommendation algorithms or libraries, depending on the application’s needs.
- Data Security: Handling data in-house (as opposed to third-party tools) provides greater control over user data.
- Real-time Updates: Recommendations can be updated in real-time as user data changes, offering fresher suggestions.
Conclusion
Creating personalized experiences is essential for retaining users and enhancing their interaction with your platform. Django, with its rich ecosystem and Python’s extensive library support, is an excellent tool for integrating recommendation systems into web applications. By understanding the fundamentals and implementing them, developers can drive user engagement and satisfaction.
Table of Contents
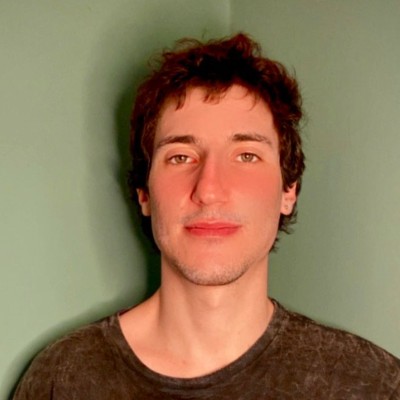
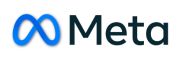