How to set up caching?
Setting up caching in Django can significantly improve the performance and responsiveness of your web application by reducing the need to regenerate data or templates for each user request. Django offers a robust and flexible caching framework that allows you to cache various components of your application. Here’s a step-by-step guide on how to set up caching in Django:
- Configure Caching Backend:
In your project’s settings (usually `settings.py`), start by configuring the caching backend. Django supports various caching backends, including in-memory caches like Memcached and file-based caches. Here’s an example using Memcached:
```python CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.memcached.MemcachedCache', 'LOCATION': '127.0.0.1:11211', # Replace with your Memcached server details } } ```
You can choose the caching backend that best suits your application’s needs.
- Enable Caching Middleware:
To cache the entire page or specific views, you can enable caching middleware in your project’s settings:
```python MIDDLEWARE = [ # ... 'django.middleware.cache.UpdateCacheMiddleware', # Add before CommonMiddleware 'django.middleware.common.CommonMiddleware', 'django.middleware.cache.FetchFromCacheMiddleware', # Add after CommonMiddleware # ... ] ```
- Cache Decorators:
Django provides decorators that allow you to cache the output of specific views. For example, you can use the `cache_page` decorator to cache the result of a view for a specific duration:
```python from django.views.decorators.cache import cache_page @cache_page(60 * 15) # Cache for 15 minutes def my_view(request): # Your view logic here ```
- Template Fragment Caching:
You can also cache specific parts of a template using the `{% cache %}` template tag. This is useful for caching dynamic portions of a template while rendering the rest dynamically.
- Low-Level Caching API:
Django’s low-level caching API allows you to cache arbitrary data, such as database query results or expensive computations. You can use functions like `cache.set()` and `cache.get()` to store and retrieve data from the cache.
- Cache Invalidation:
It’s essential to plan cache invalidation strategies to ensure that cached data remains up to date. You can manually invalidate cache keys or use the `cache.clear()` method to clear the entire cache.
By following these steps, you can effectively set up caching in Django to optimize your application’s performance and reduce server load. Caching can dramatically improve response times, especially for content that doesn’t change frequently, making your web application more efficient and responsive to user requests.
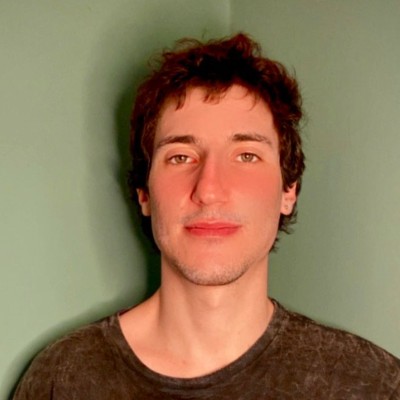
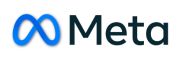