Django and Single Sign-On: Implementing SSO in Your Web App
In the ever-evolving landscape of web development, user authentication and security are paramount. Single Sign-On (SSO) is a powerful solution that can not only enhance the security of your web application but also provide a seamless user experience. In this blog post, we will explore how to implement SSO in your Django web app.
Table of Contents
1. What is Single Sign-On (SSO)?
Single Sign-On (SSO) is an authentication process that allows a user to access multiple applications with a single set of login credentials. Instead of having to remember multiple usernames and passwords for different services, users can log in once and gain access to various applications seamlessly. This not only simplifies the user experience but also enhances security by reducing the need for users to remember multiple passwords.
2. Benefits of SSO
Implementing SSO in your Django web app can offer several benefits, including:
- Enhanced Security: SSO centralizes authentication, making it easier to enforce security policies and monitor user access. It reduces the risk of password-related security breaches.
- Improved User Experience: Users appreciate the convenience of logging in once and accessing multiple services. This can lead to higher user satisfaction and engagement.
- Simplified Management: Managing user accounts and access becomes more efficient since it’s centralized, reducing administrative overhead.
- Integration Flexibility: SSO can be integrated with various authentication providers, including social media logins, LDAP, and SAML, giving you flexibility in how you authenticate users.
Now, let’s dive into implementing SSO in your Django web app step by step.
Step 1: Choose an SSO Provider
The first step in implementing SSO is choosing an SSO provider. An SSO provider is a service that handles user authentication and provides a way for your application to trust the authentication process. Some popular SSO providers include Okta, Auth0, Google, and Microsoft Azure Active Directory.
Example: Using Google as an SSO Provider
For this example, we’ll use Google as the SSO provider. You’ll need to set up a project in the Google Cloud Console and configure OAuth 2.0 credentials. Make sure to note the client ID and client secret, as you’ll need them later.
Step 2: Install Required Libraries
To integrate SSO into your Django web app, you’ll need to install some Python libraries. The two most commonly used libraries are python-social-auth and social-auth-app-django.
bash pip install python-social-auth social-auth-app-django
Step 3: Configure Django Settings
Next, you’ll need to configure your Django settings to work with the chosen SSO provider. Open your project’s settings.py file and add the following configurations:
python # settings.py AUTHENTICATION_BACKENDS = ( 'social_core.backends.google.GoogleOAuth2', # Replace with your SSO provider 'django.contrib.auth.backends.ModelBackend', ) SOCIAL_AUTH_GOOGLE_OAUTH2_KEY = 'your-client-id' SOCIAL_AUTH_GOOGLE_OAUTH2_SECRET = 'your-client-secret' LOGIN_URL = 'login' LOGOUT_URL = 'logout' LOGIN_REDIRECT_URL = 'home'
Replace ‘social_core.backends.google.GoogleOAuth2’ with the appropriate backend for your SSO provider.
Step 4: Create URLs and Views
Now, you’ll need to create URLs and views for handling SSO-related actions, such as login and logout. In your Django project’s urls.py file, add the following URL patterns:
python # urls.py from django.urls import path, re_path, include urlpatterns = [ path('auth/', include('social_django.urls', namespace='social')), # Your other URL patterns ]
Create views for handling login and logout as well. Here’s an example:
python # views.py from django.shortcuts import render, redirect from django.contrib.auth import logout as auth_logout def logout(request): """Logout user.""" auth_logout(request) return redirect('home') # Your other views
Step 5: Create Templates
Create templates for the login and logout pages. These templates should include buttons or links for users to initiate the SSO process and log out. Customize the templates to match your application’s design and branding.
html <!-- login.html --> <!DOCTYPE html> <html> <head> <title>Login</title> </head> <body> <h1>Login</h1> <a href="{% url 'social:begin' 'google-oauth2' %}">Login with Google</a> </body> </html> html <!-- logout.html --> <!DOCTYPE html> <html> <head> <title>Logout</title> </head> <body> <h1>Logout</h1> <a href="{% url 'logout' %}">Logout</a> </body> </html>
Step 6: Test Your SSO Integration
With everything set up, you can now test your SSO integration. Start your Django development server and access the login page. You should see a “Login with Google” button. Clicking this button should initiate the SSO process and redirect you to the Google login page. After successful authentication, you’ll be redirected back to your web app.
Step 7: Customize User Data Sync
By default, the SSO integration will create a new user in your Django application for each authenticated SSO user. You can customize how user data is synced by creating a custom pipeline.
python # settings.py SOCIAL_AUTH_PIPELINE = ( # ... 'yourapp.pipeline.sync_user_data', # Create this function # ... )
In your custom pipeline function, you can define how user data is extracted from the SSO provider and saved to your Django user model.
Step 8: Handle User Logout
Implementing user logout is equally important. Users should have the option to log out of your application securely. You’ve already created the logout view in Step 4. Make sure to include a logout button or link in your application’s UI, which should redirect users to the logout view.
Step 9: Implement Access Control
To control access to specific views or resources within your Django web app, you can use Django’s built-in @login_required decorator. For example:
python # views.py from django.contrib.auth.decorators import login_required from django.shortcuts import render @login_required def protected_view(request): """A view that requires authentication.""" return render(request, 'protected.html')
By using @login_required, you ensure that only authenticated users can access the protected_view.
Conclusion
Implementing Single Sign-On (SSO) in your Django web app can greatly enhance security and user convenience. By choosing an SSO provider, configuring Django settings, and creating the necessary views and templates, you can seamlessly integrate SSO into your application. Additionally, customizing user data synchronization and access control allows you to tailor the SSO experience to your specific requirements.
Enhance the authentication experience for your users and boost the security of your Django web app by implementing SSO today. With the right SSO provider and these steps, you’ll be well on your way to a more streamlined and secure authentication process.
Table of Contents
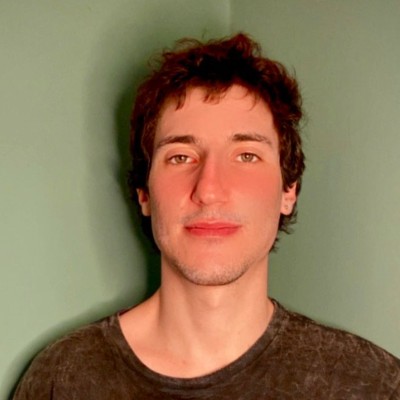
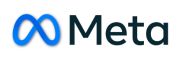