How to handle user authentication and permissions in a Django REST framework API?
Handling user authentication and permissions in a Django REST framework (DRF) API is crucial for securing your API endpoints and ensuring that only authorized users can perform certain actions. Here’s a comprehensive approach to managing user authentication and permissions in a DRF API:
- Authentication:
– Use DRF’s built-in authentication classes like `TokenAuthentication` or `SessionAuthentication` to enable user authentication. `TokenAuthentication` is commonly used for stateless authentication via tokens, while `SessionAuthentication` relies on session cookies for stateful authentication.
- User Registration and Login:
– Implement user registration and login views to allow users to create accounts and obtain authentication tokens or use username/password-based login.
- Permissions:
– Define permissions for your views. DRF provides various permission classes like `IsAuthenticated`, `IsAuthenticatedOrReadOnly`, and custom permissions. Assign permissions to views based on the desired level of access control.
- User Roles and Groups:
– Assign users to roles or groups using Django’s built-in mechanisms. Create custom permissions if necessary to fine-tune access control within your API.
- Serializer Validation:
– Ensure that your serializers validate user input and check permissions when creating, updating, or deleting resources. You can override serializer methods like `create()` and `update()` to implement custom permission checks.
- Token-Based Authentication:
– Use token-based authentication (e.g., JSON Web Tokens or JWTs) for added security and scalability. DRF offers packages like `djangorestframework-jwt` for JWT support.
- View Decorators:
– Utilize DRF’s view decorators like `@permission_classes` and `@authentication_classes` to apply authentication and permission checks to specific views or viewsets.
- Error Handling:
– Implement proper error handling for unauthorized access. Return appropriate HTTP status codes (e.g., 401 Unauthorized or 403 Forbidden) and informative error messages.
- Logging and Auditing:
– Implement logging and auditing to track user actions and monitor API access for security purposes.
- Password Management:
– Implement password reset and change functionality to enhance security and user experience.
By following these best practices, you can effectively handle user authentication and permissions in your DRF API. This ensures that your API remains secure, accessible only to authorized users, and adheres to your application’s access control requirements.
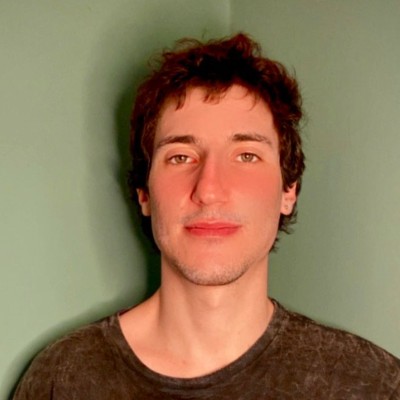
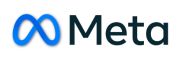