Django Q & A
How to handle user authentication using third-party providers like Google or Facebook in Django?
Handling user authentication using third-party providers like Google or Facebook in Django involves integrating their authentication services into your application. Here’s a step-by-step guide on how to achieve this:
- Choose an Authentication Provider: Decide which third-party authentication provider you want to use. Popular choices include Google, Facebook, Twitter, and GitHub. Each provider has its own developer portal where you can set up authentication.
- Create Developer Accounts: Sign up for a developer account with the chosen authentication provider. This will give you access to the necessary credentials and settings for integration.
- Set Up Your Application: Register your Django application with the third-party provider. You’ll typically need to provide details like your application’s name, callback URL (where users are redirected after authentication), and other settings.
- Obtain API Credentials: Obtain API credentials (Client ID and Client Secret) from the provider’s developer portal. These credentials are required to authenticate your application with the third-party service.
- Install Required Packages: Install Django packages that simplify third-party authentication, such as `python-social-auth`, `django-allauth`, or `django-rest-auth` (for REST APIs).
- Configure Django Settings: Configure your Django settings to include the credentials and settings provided by the third-party provider. You’ll typically find places for these configurations in your `settings.py` file. Make sure to add your client ID and client secret here.
- Set Up URLs and Views: Define URL patterns and views in your Django application for handling authentication. You may need to create a callback view that captures the authentication response from the third-party provider.
- User Mapping: Determine how user accounts will be created and managed in your Django application. You can either link third-party accounts to existing user profiles or create new user accounts upon successful authentication.
- Testing: Thoroughly test the authentication flow. Ensure that users can log in using their third-party accounts and that user data is correctly mapped and synchronized with your Django database.
- User Experience: Provide a seamless user experience by customizing login and registration templates and ensuring clear messaging during the authentication process.
- Security Considerations: Implement security best practices, such as verifying tokens, protecting against CSRF attacks, and securely storing API credentials.
- Documentation: Document the integration process and settings for your team and future developers who may work on the project.
By following these steps, you can successfully implement user authentication using third-party providers like Google or Facebook in your Django application, offering users a convenient and secure way to access your services.
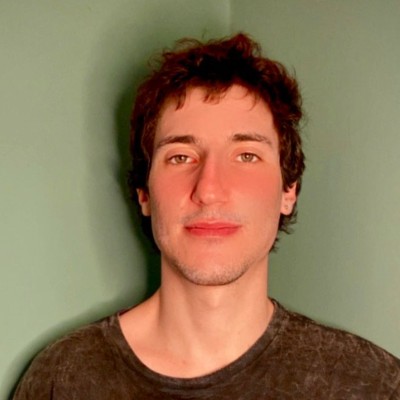
Previously at
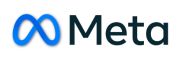
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.