Django Q & A
How to implement a user rating and review system in Django?
Implementing a user rating and review system in Django can greatly enhance user engagement and feedback for your application. Here’s a step-by-step guide on how to achieve this:
- Database Models: Start by creating database models to store ratings and reviews. You’ll typically need two models, one for ratings and another for reviews. The ratings model can have fields like `user`, `item` (e.g., a product or service being reviewed), and the `rating` value (usually a number from 1 to 5). The reviews model should include fields for `user`, `item`, `text` (the review content), and a timestamp.
- User Authentication: Ensure that user authentication is set up in your Django project, as you’ll need to associate ratings and reviews with specific users. Django’s built-in authentication system can be used for this purpose.
- Views and Forms: Create views for users to submit ratings and reviews. You may also want to create forms for this purpose. Use Django’s form handling and validation capabilities to collect user input.
- Templates: Design templates for displaying ratings and reviews. You can include star ratings, user avatars, and review text. Use Django’s templating engine to render this information dynamically.
- Business Logic: Implement the business logic for calculating average ratings for items. You can do this by querying the database for ratings associated with a particular item and then calculating the average rating.
- Display Ratings and Reviews: Display ratings and reviews on your website or app wherever relevant. You can display average ratings alongside items in listings and individual ratings and reviews on item detail pages.
- Sorting and Filtering: Provide options for users to sort and filter items based on ratings or recency of reviews. Django’s query capabilities can help you filter and order items accordingly.
- User Interaction: Allow users to update their ratings and reviews and provide options for liking or disliking reviews. You may also want to implement moderation features to manage inappropriate content.
- Notifications: Implement a notification system to inform users when someone interacts with their reviews, e.g., liking or commenting on them.
- Security: Ensure that your system is secure and protected against spam or fake reviews. Implement CAPTCHA or other anti-bot measures if necessary.
- Testing: Write unit and integration tests for your rating and review system to ensure that it functions correctly and reliably.
- Scalability: Consider how your system will scale as the number of reviews and ratings grows. Optimize database queries and caching for better performance.
- API Endpoints (Optional): If your application has an API, create endpoints to allow programmatic access to ratings and reviews, which can be useful for third-party integrations.
- Documentation: Document the usage of your rating and review system for developers and users, including any API documentation if applicable.
By following these steps, you can successfully implement a user rating and review system in your Django application. This system can enhance user engagement, provide valuable feedback, and improve the overall user experience on your platform.
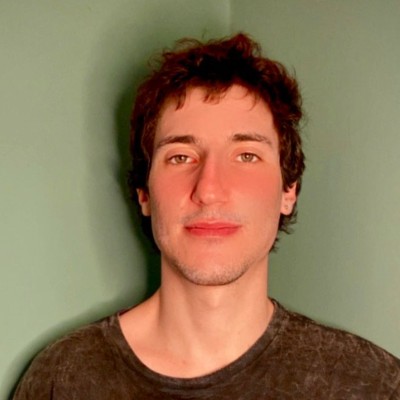
Previously at
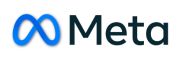
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.