When Web Development Meets Virtual Reality: The Django Approach
The world of web development has come a long way with the advent of powerful backend frameworks like Django. Meanwhile, Virtual Reality (VR) is taking the world by storm, providing immersive experiences for users in gaming, education, real estate, and many other industries. One might wonder: Can Django, known for building robust web applications, be used to power VR experiences? The answer is a surprising “yes”.
Table of Contents
In this blog post, we will delve into the symbiotic relationship between Django and Virtual Reality, showing you how you can integrate Django with VR platforms and build unparalleled immersive experiences.
1. Why Django?
At its core, Django is a high-level web framework that encourages rapid development and clean, pragmatic design. Built by experienced developers, Django handles much of the web development process’s hassle, allowing you to focus on writing your app without reinventing the wheel.
While Django might not be the first thing you think of when considering VR, its features can greatly complement a VR setup:
- Admin Interface: Easily manage VR content, user profiles, and experiences.
- Database Models: Organize and store VR assets, user progress, and more.
- RESTful API: Integrate with VR platforms or engines, serving data and assets on-demand.
2. Introducing A-Frame: A WebVR Framework
For this discussion, we’ll be looking at A-Frame, an open-source WebVR framework. A-Frame allows developers to build VR experiences using simple HTML, making it incredibly accessible for web developers.
3. Building a Simple VR Scene with Django and A-Frame
Step 1: Set Up Your Django Project
First, let’s start a new Django project and a new app:
```bash django-admin startproject vr_project cd vr_project python manage.py startapp vr_app ```
Step 2: A-Frame Integration
In your Django app’s templates, let’s create a new file called `vr_scene.html`. Here’s how you can set up a basic VR scene using A-Frame:
```html <!DOCTYPE html> <html> <head> <title>Simple VR Scene</title> <script src="https://aframe.io/releases/1.2.0/aframe.min.js"></script> </head> <body> <a-scene> <a-box position="-1 0.5 -3" rotation="0 45 0" color="#4CC3D9"></a-box> <a-sphere position="0 1.25 -5" radius="1.25" color="#EF2D5E"></a-sphere> <a-cylinder position="1 0.75 -3" radius="0.5" height="1.5" color="#FFC65D"></a-cylinder> <a-plane position="0 0 -4" rotation="-90 0 0" width="4" height="4" color="#7BC8A4"></a-plane> <a-sky color="#ECECEC"></a-sky> </a-scene> </body> </html> ```
Step 3: Serving Dynamic VR Content
Imagine you want your VR objects (box, sphere, etc.) to be stored in a Django model and served dynamically. Here’s a simplified model:
```python from django.db import models class VRObject(models.Model): SHAPE_CHOICES = [ ('box', 'Box'), ('sphere', 'Sphere'), ('cylinder', 'Cylinder'), ] shape = models.CharField(choices=SHAPE_CHOICES, max_length=10) position = models.CharField(max_length=50) rotation = models.CharField(max_length=50) color = models.CharField(max_length=7) ```
You can then use Django’s ORM capabilities to retrieve these objects from the database and render them dynamically in the VR scene.
```python # views.py from django.shortcuts import render from .models import VRObject def vr_scene(request): vr_objects = VRObject.objects.all() return render(request, 'vr_scene.html', {'vr_objects': vr_objects}) ```
In `vr_scene.html`, iterate over the VR objects:
```html <a-scene> {% for obj in vr_objects %} <a-{{ obj.shape }} position="{{ obj.position }}" rotation="{{ obj.rotation }}" color="{{ obj.color }}"></a-{{ obj.shape }}> {% endfor %} <a-sky color="#ECECEC"></a-sky> </a-scene> ```
3. Beyond Basics: Complex VR Experiences
The above example scratches the surface. With Django’s capabilities:
– Use the Django REST framework to build APIs serving complex VR experiences.
– Implement user authentication to provide personalized VR experiences.
– Use Django Channels for real-time multiplayer VR experiences.
Conclusion
While Django wasn’t originally designed with VR in mind, its robustness, scalability, and adaptability make it a powerful tool for those looking to experiment in the intersection of web development and VR. By understanding the basics of integrating Django with VR frameworks like A-Frame, developers can usher in a new era of immersive web-based VR experiences.
Table of Contents
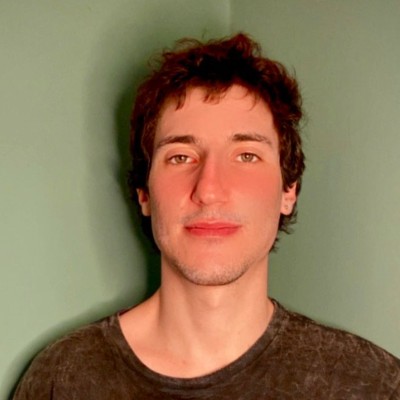
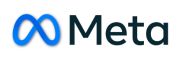