Unlocking Elixir’s Potential: A Guide to Advanced Techniques and Best Practices
Elixir is a dynamic, functional language developed for building scalable and maintainable applications. With the growing demand for this efficient language, many companies decide to hire Elixir developers to maximize the use of this technology. The language runs on the Erlang virtual machine (BEAM), inheriting many benefits including lightweight process threads, low-latency real-time distribution, and fault-tolerance. Today, we will delve into some advanced tips and tricks in Elixir that not only will help you master its power, but also provide insights into what you should look for when you hire Elixir developers.
1. Pattern Matching
One of the fundamental features in Elixir is pattern matching, which serves as an alternative to variable assignment in other languages. In Elixir, the `=` operator matches the left-hand side with the right-hand side, and binds variables when a successful match is found.
For instance:
```elixir {a, b, c} = {:hello, "world", 42} ```
This binds `a` to `:hello`, `b` to `”world”`, and `c` to `42`.
1.1 Using `_` as an Ignore
When pattern matching, you may want to ignore some values. For this, Elixir provides `_`.
```elixir {a, _, c} = {:hello, "world", 42} ```
In this case, `”world”` is ignored, and `a` and `c` are bound to `:hello` and `42` respectively.
2. Functions
2.1 Default Arguments and Pattern Matching
Functions in Elixir can have default arguments. These can work together with pattern matching for powerful results.
```elixir defmodule Math do def add(a, b \\ 0) do a + b end end ```
The function `add` can now be called with either one or two arguments.
2.2 Guard Clauses
Guard clauses are a way of enhancing pattern matching in function definitions. They add additional rules, expressed as boolean expressions, that must be met for a function clause to match.
```elixir defmodule Math do def absolute(n) when n >= 0 do n end def absolute(n) when n < 0 do -n end end ```
The function `absolute` will now return the absolute value of `n`, thanks to the guard clauses.
3. Metaprogramming and Macros
Elixir’s metaprogramming capabilities are some of its most powerful features. Metaprogramming in Elixir is done using macros, which are a way to write code that writes other code during compile time.
Here’s a simple example of a macro:
```elixir defmodule Logger do defmacro log(msg) do quote do IO.puts("Logging: #{unquote(msg)}") end end end ```
You can use it in your code like so:
```elixir require Logger Logger.log("This is a test message") ```
The above code will output: `Logging: This is a test message`.
4. Advanced Data Structures: Maps and Structs
Elixir provides a robust map data structure, which is a key-value store that offers constant-time reads and writes.
```elixir map = %{:foo => "bar", "hello" => :world} IO.puts(map[:foo]) # => "bar" IO.puts(map["hello"]) # => "world" ```
Structs are a special kind of map that allow for default values and compile-time checks.
```elixir defmodule User do defstruct name: "John Doe", age: 27 end jane = %User{name: "Jane Doe"} IO.puts(jane.name) # => "Jane Doe" IO.puts(jane.age) # => 27 ```
5. Asynchronous Programming with Tasks
Elixir has first-class support for concurrent and distributed programming with its `Task` module.
```elixir defmodule Example do def run do task = Task.async(fn -> perform_complex_calculation() end) # Do other work result = Task.await(task) # Use the result end end ```
In the `run` function, `Task.async` starts a new process that executes `perform_complex_calculation()`. The main process can continue to do other work. When the result of the calculation is needed, `Task.await` is called. If the calculation is done, it returns the result; if not, it waits until the result is available.
6. GenServer and OTP
One of the features that make Elixir powerful for building distributed systems is its OTP (Open Telecom Platform) library. GenServer, a behavior module for implementing the server of a client-server relation, is a part of OTP. Understanding these aspects of Elixir is essential, especially when you’re planning to hire Elixir developers. Their expertise in these areas can significantly boost the performance and scalability of your applications.
```elixir defmodule Stack do use GenServer # Callbacks def handle_call(:pop, _from, [head | tail]) do {:reply, head, tail} end def handle_call({:push, element}, _from, stack) do {:reply, :ok, [element | stack]} end end ```
In the above code, the `Stack` module uses GenServer and defines two callback functions. When the server receives a `:pop` message, it responds with the head of the stack and updates its state to the tail of the stack. When it receives a `{:push, element}` message, it responds with `:ok` and pushes the element onto the stack.
Conclusion
These examples only scratch the surface of what you can achieve with Elixir. Its powerful features, combined with the robustness of the Erlang VM, make it an excellent choice for building concurrent and distributed systems. If these complexities seem daunting, you might consider the option to hire Elixir developers. Whether you’re just getting started, looking to enhance an existing project, or wishing to onboard expert help, these advanced tips and tricks along with professional assistance should undoubtedly help you level up your Elixir skills.
Table of Contents
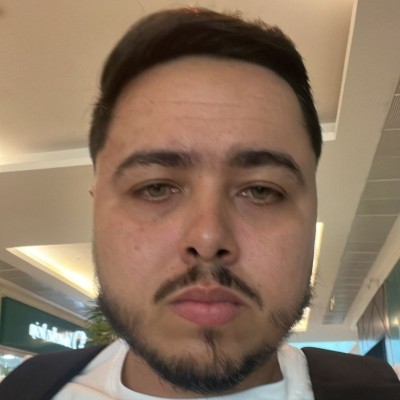
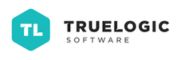