Embarking on the Elixir Journey: A Comprehensive Beginner’s Guide to Getting Started
Elixir is an impressive language that has been making waves in the world of software development, so much so that businesses are now looking to hire Elixir developers more than ever. Its benefits are undeniable: scalable, maintainable, and concurrent programming abilities make it a top pick for various applications, especially those related to the web.
In this beginner’s guide, we will provide a comprehensive introduction to this exciting language. This is not only crucial for those who want to learn and master Elixir, but also for companies that aim to hire Elixir developers, as it will help them understand what skills to look for.
Whether you are entirely new to programming, an experienced coder venturing into a new language, or a company seeking to hire Elixir developers, this guide should give you a solid foundation on which to build your Elixir knowledge.
1. Introduction to Elixir
Elixir is a dynamic, functional language designed for building scalable and maintainable applications. José Valim, a Ruby enthusiast, created it to address some concerns about Ruby’s concurrency and performance. Leveraging the Erlang VM, known for running low-latency, distributed, and fault-tolerant systems, Elixir provides productive tooling and an extensible design perfect for web development and the software industry at large.
2. Setting Up Your Environment
The first step in starting your journey with Elixir is setting up your environment. You can install Elixir on macOS, Windows, or Linux. Visit the Elixir-Lang website for a step-by-step installation guide.
Once installed, you can verify the installation by opening your terminal and typing `elixir -v`. You should see something like this:
``` Elixir 1.10.3 (compiled with Erlang/OTP 22) ```
Now that you have your environment ready, let’s delve into the Elixir syntax and semantics.
3. Basic Syntax and Semantics
Elixir’s syntax is clean, concise, and friendly to beginners. It borrows some syntactic elements from Ruby, making it easy to read and write.
3.1 Hello World
As with any programming language tutorial, we’ll start with a ‘Hello, World!’ example. Open your favorite text editor, create a new file called `hello.exs` and type the following:
```elixir IO.puts "Hello, World!" ```
Save your file and run it using the `elixir` command in your terminal:
``` elixir hello.exs ```
You should see `Hello, World!` printed to your terminal.
3.2 Variables
Variables in Elixir are dynamically typed and easy to declare. Here’s an example:
```elixir name = "Alice" IO.puts name ```
3.3 Functions
Elixir is a functional programming language, meaning that functions are first-class citizens. Here’s how you can define a simple function:
```elixir defmodule Math do def add(a, b) do a + b end end IO.puts Math.add(5, 7) # prints 12 ```
In this example, `defmodule` is used to define a module called `Math`. Inside the module, we defined a function `add` that takes two parameters and returns their sum.
3.4 Control Structures
Elixir also provides familiar control structures such as `if`, `case`, and `cond`.
```elixir # if control structure if true do IO.puts "This will be printed" end # case control structure case 1 do 1 -> IO.puts "One" 2 -> IO.puts "Two" _ -> IO.puts "Not One or Two" end # cond control structure cond do 2 + 2 == 5 -> "This will not be seen" 2 * 2 == 3 -> "Nor this" true -> "But this will" end ```
3.5 Pattern Matching
One of Elixir’s unique features is pattern matching, which is not a common feature in most mainstream languages.
```elixir # Pattern matching with tuples {a, b, c} = {:hello, "world", 42} IO.puts a # prints :hello IO.puts b # prints "world" IO.puts c # prints 42 ```
In this example, we match the tuple on the left with the one on the right. As a result, `a` gets the value `:hello`, `b` gets `”world”`, and `c` gets `42`.
4. Concurrency
Elixir excels at building concurrent applications thanks to the Erlang VM. Here’s a simple example of spinning up multiple Elixir processes:
```elixir defmodule Worker do def start do spawn_link(fn -> perform_work() end) end defp perform_work do IO.puts "Work, work!" :timer.sleep(1000) perform_work() end end # spawn two workers Worker.start() Worker.start() ```
In this code, `spawn_link` creates a new process that executes the given function. The function `perform_work` prints a message and then calls itself after a delay, creating a loop.
Conclusion
This article provided an introduction to Elixir, a language that is increasingly in demand as businesses look to hire Elixir developers. We covered its installation, basic syntax, functions, control structures, pattern matching, and concurrency. The simplicity and power of Elixir, coupled with its scalability and concurrency features, make it not only an exciting language for developers to learn, but also a compelling reason for companies to hire Elixir developers.
Remember, this is just a basic overview. Elixir boasts many more advanced features such as metaprogramming, polymorphism, and robust error handling that are beyond the scope of this beginner’s guide but will certainly be relevant to those aiming to hire Elixir developers.
The journey of mastering Elixir, like any other programming language, involves practice and continuous learning. Whether you are an aspiring developer or a company looking to hire Elixir developers, the basics covered in this guide should get you started. Keep experimenting, keep learning, and enjoy the journey!
Table of Contents
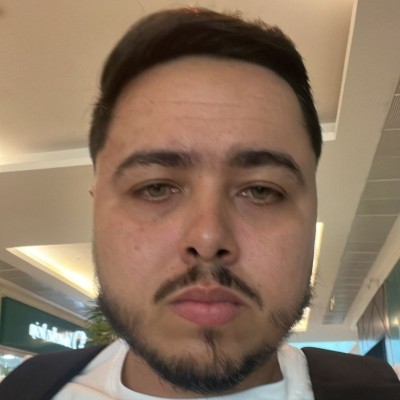
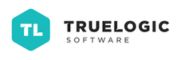