Exploring Elixir’s Built-in Testing Framework: ExUnit
In the bustling world of Elixir, where productivity meets scalability, testing is not just a box to tick; it’s a fundamental aspect of the development process. Enter ExUnit, Elixir’s built-in testing framework, designed to streamline the process of writing and executing tests while ensuring the robustness of your codebase.
1. Understanding ExUnit
ExUnit embodies the Elixir philosophy of simplicity and power. It provides developers with a cohesive set of tools to write comprehensive tests for their applications. At its core, ExUnit follows the xUnit style of testing, which structures tests into modules and functions, making it intuitive for developers familiar with other testing frameworks.
2. Writing Your First Test
Let’s dive right in with a simple example. Suppose we have a module called `Math` with a function `add/2` that adds two numbers together. Here’s how we would write a test for it using ExUnit:
```elixir defmodule MathTest do use ExUnit.Case test "add/2 adds two numbers together" do assert Math.add(1, 2) == 3 end end ```
In this test module, we use `ExUnit.Case` to define our test case. Inside the `test` macro, we make an assertion using `assert`, ensuring that the result of `Math.add(1, 2)` equals `3`.
3. Running Tests
Running tests in Elixir is as simple as running a single command in your terminal:
```bash mix test ```
This command will automatically discover and execute all the test modules in your project, providing detailed feedback on the success or failure of each test.
4. Harnessing the Power of ExUnit
ExUnit goes beyond the basics, offering a plethora of features to enhance your testing experience.
5. Test Setup and Teardown
Often, tests require certain preconditions to be met before they can be executed or cleanup actions to be performed afterward. ExUnit provides `setup` and `teardown` callbacks for precisely these purposes:
```elixir defmodule DatabaseTest do use ExUnit.Case setup do # Set up database connection {:ok, conn: MyApp.Database.start_link} end test "fetching data from the database" do assert MyApp.Database.fetch_data() == expected_data end teardown %{conn: conn} do # Close database connection :ok = MyApp.Database.stop(conn) end end ```
6. Test Exclusions and Tags
With ExUnit, you can selectively run or exclude tests based on tags. This feature is particularly useful for isolating specific test suites or excluding tests that are currently failing:
```elixir defmodule MyTest do use ExUnit.Case, async: true @tag :slow test "slow test" do # Some slow test case end @tag :pending test "pending test" do # Some pending test case end end ```
Running tests with specific tags:
```bash mix test --include slow ```
7. Mocking and Stubbing
ExUnit provides utilities for mocking and stubbing external dependencies, allowing you to isolate units of code under test and simulate various scenarios:
```elixir defmodule MyTest do use ExUnit.Case test "mocking HTTP requests" do HTTPMock.expect(:get, "http://example.com", fn(_) -> {:ok, "Mocked response"} end) assert MyApp.fetch_data() == "Mocked response" end end ```
Conclusion
ExUnit empowers Elixir developers to write expressive, reliable tests with ease. Its intuitive syntax, coupled with powerful features like test setup, tags, and mocking, makes it a formidable ally in the quest for code quality and reliability.
So why wait? Dive into the world of ExUnit today and elevate your Elixir testing game to new heights!
External Resources:
- Official ExUnit Documentation – https://hexdocs.pm/ex_unit/ExUnit.html
- Elixir School – Testing – https://elixirschool.com/en/lessons/basics/testing/
- Mocking in Elixir with Mox – https://dashbit.co/blog/mocking-in-elixir-with-mox
Table of Contents
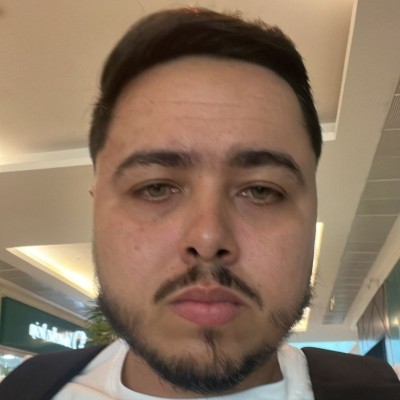
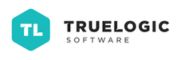