Caching Strategies in Elixir: Techniques and Best Practices
In the realm of modern software development, optimizing performance is a critical aspect. As applications grow in complexity and data volumes increase, efficiently managing data retrieval and processing becomes essential. One powerful approach to achieve this optimization is through caching. Caching involves storing frequently accessed data in a temporary storage layer, which significantly reduces the need to fetch the data from the original source repeatedly. In this blog, we’ll delve into caching strategies in the context of Elixir, a functional and concurrent programming language known for its scalability and fault-tolerance.
Table of Contents
1. Why Caching Matters in Elixir
Before diving into the techniques and best practices, it’s crucial to understand why caching is relevant in the Elixir ecosystem. Elixir, built on the Erlang virtual machine, excels in building highly concurrent and fault-tolerant systems. While its processes are lightweight, they can still become bottlenecks if they perform computationally intensive tasks or interact with slow external resources like databases or APIs. Caching mitigates these bottlenecks by storing frequently accessed data, reducing the load on these processes and improving overall system performance.
2. Common Caching Scenarios
Caching can be applied to various scenarios in an Elixir application. Let’s explore some common scenarios where caching can provide significant benefits:
2.1. Database Query Results:
Database queries are often a source of latency. By caching query results, you can avoid hitting the database repeatedly for the same data. Consider the following code snippet:
elixir defmodule UserService do @ttl 3600 # Cache time-to-live in seconds def get_user(id) do case Cache.get("user_#{id}") do nil -> user = Database.get_user(id) Cache.put("user_#{id}", user, ttl: @ttl) user cached_user -> cached_user end end end
In this example, the get_user/1 function first checks the cache for the user’s data. If the data is not found in the cache, it fetches the user from the database, stores it in the cache, and returns it. Subsequent calls within the cache’s time-to-live will directly retrieve the user data from the cache.
2.2. Expensive Calculations:
Elixir’s concurrency model allows for parallel execution of tasks. However, some calculations might still be computationally expensive. Caching the results of these calculations can save processing time. Here’s an illustration:
elixir defmodule MathService do @ttl 1800 # Cache time-to-live in seconds def calculate_fibonacci(n) do case Cache.get("fibonacci_#{n}") do nil -> result = compute_fibonacci(n) Cache.put("fibonacci_#{n}", result, ttl: @ttl) result cached_result -> cached_result end end defp compute_fibonacci(0), do: 0 defp compute_fibonacci(1), do: 1 defp compute_fibonacci(n), do: compute_fibonacci(n - 1) + compute_fibonacci(n - 2) end
In this scenario, the calculate_fibonacci/1 function uses caching to store previously computed Fibonacci values, reducing redundant computations.
3. Choosing the Right Cache Store
Elixir offers various caching libraries and stores to choose from, each with its own advantages and limitations. Let’s explore some popular options:
3.1. ETS (Erlang Term Storage):
ETS is an in-memory store built into the Erlang runtime. It offers blazing-fast access times and is highly concurrent. It’s suitable for scenarios where you need very low-latency caching and can store the data entirely in memory. However, ETS data is volatile and can be lost if the system crashes or restarts.
elixir :ets.new(:my_cache, [:public, :named_table]) :ets.insert(:my_cache, {:key, value}) {:ok, value} = :ets.lookup(:my_cache, :key)
3.2. Cachex:
Cachex is a popular Elixir caching library that provides a flexible API and supports various storage backends, including ETS, Redis, and others. This flexibility allows you to adapt the caching strategy based on your application’s requirements.
To use Cachex, add it to your mix.exs:
elixir defp deps do [{:cachex, "~> 3.0"}] end
Then, configure Cachex and start using it in your application:
elixir Cachex.start_link() Cachex.put(:my_cache, :key, value) value = Cachex.get(:my_cache, :key)
3.3. Redis:
Redis is a highly popular in-memory data store that can be used as a caching layer. It offers persistence and can be configured for various eviction policies. The Redix library provides an Elixir client for Redis.
To use Redix, add it to your mix.exs:
elixir defp deps do [{:redix, "~> 0.11"}] end
Then, interact with Redis using Redix:
elixir {:ok, conn} = Redix.start_link() :ok = Redix.command(conn, ["SET", "key", "value"]) {:ok, value} = Redix.command(conn, ["GET", "key"])
4. Cache Invalidation Strategies
While caching provides performance benefits, it’s crucial to manage cache invalidation effectively to ensure that the cached data remains accurate. Stale or outdated data can lead to incorrect application behavior. Here are some cache invalidation strategies:
4.1. Time-Based Expiration:
In this strategy, cached items are assigned a fixed time-to-live (TTL). Once the TTL expires, the cache automatically removes the item. This is useful for scenarios where data updates are infrequent.
4.2. Event-Based Invalidation:
Instead of relying solely on a fixed TTL, you can invalidate cache items when relevant data changes occur. This can be achieved using message passing between processes or through Elixir’s built-in PubSub mechanism.
4.3. Manual Invalidation:
For fine-grained control, you can invalidate cache items explicitly in response to specific events or changes. This gives you full control over when and what to invalidate.
Conclusion
Caching strategies are an essential tool for optimizing the performance of Elixir applications. By intelligently caching frequently accessed data, you can reduce resource-intensive operations, improve response times, and enhance the overall user experience. Whether you’re dealing with database query results, expensive calculations, or other data-intensive tasks, adopting effective caching strategies can lead to a more responsive and scalable application. Explore the caching libraries available in Elixir, choose the right caching store for your use case, and implement appropriate cache invalidation strategies to keep your data accurate and up-to-date. With these techniques and best practices, you’ll be well-equipped to harness the power of caching and elevate your Elixir applications to new heights of performance and efficiency.
Table of Contents
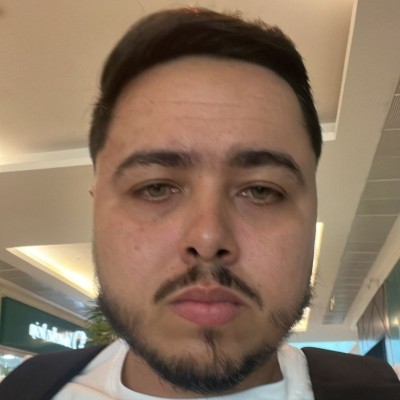
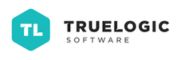