Building a Real-Time Chat Application with Elixir and WebSockets
In today’s fast-paced digital landscape, real-time communication has become the backbone of numerous applications, from messaging platforms to collaborative tools. Asynchronous messaging protocols like WebSockets have revolutionized how we interact online, enabling seamless, instant communication between users. In this guide, we’ll delve into building a real-time chat application using the power of Elixir and WebSockets.
1. Elixir and WebSockets
Elixir, a functional programming language built on the Erlang VM, is renowned for its scalability, fault tolerance, and concurrency capabilities. Combined with the robustness of WebSockets, which provides full-duplex communication channels over a single, long-lived connection, we can create highly responsive and efficient real-time applications.
2. Setting Up the Environment
Before diving into the development process, ensure you have Elixir installed on your system. You can follow the official guide https://elixir-lang.org/install.html Additionally, we’ll be using the Phoenix framework, a web development framework for Elixir, to streamline the process of building our chat application. Install Phoenix by following the instructions provided in the official documentation https://hexdocs.pm/phoenix/installation.html
3.Creating the Project Structure
Once you have Elixir and Phoenix installed, let’s kickstart our project. Open your terminal and run the following commands:
```bash mix phx.new real_time_chat --no-ecto cd real_time_chat ```
This command initializes a new Phoenix project without Ecto, the database wrapper, as we won’t be needing it for our chat application.
4. Implementing WebSockets for Real-Time Communication
With Phoenix, integrating WebSockets into our application is straightforward. Phoenix Channels, built on top of WebSockets, provide a clean abstraction for handling real-time communication. Let’s create a channel for our chat functionality:
```bash mix phx.gen.channel ChatRoom ```
This command generates the necessary files for our chat room channel, including the server-side logic and client-side JavaScript code.
5. Handling Messages in the Chat Room
Now that we have our channel set up, let’s define how messages will be handled within the chat room. We’ll leverage the Phoenix Channel’s `handle_in` function to process incoming messages and broadcast them to all connected clients. Here’s a snippet of how it’s done:
```elixir defmodule RealTimeChatWeb.ChatRoomChannel do use RealTimeChatWeb, :channel def join("chat:lobby", _params, _socket) do {:ok, "Welcome to the chat!", assign(socket, :messages, [])} end def handle_in("new_message", %{"body" => body}, socket) do broadcast socket, "new_message", %{body: body} {:noreply, assign(socket, :messages, [body | socket.assigns.messages])} end end ```
6. Building the Frontend Interface
Now that our backend logic is in place, let’s create a simple frontend interface for our chat application. We’ll use HTML, CSS, and JavaScript to render the chat messages and handle user input. You can find a basic example of the frontend code https://github.com/example/real-time-chat-app
7. Testing the Application
Before deploying our chat application, it’s crucial to thoroughly test its functionality. Utilize tools like ExUnit, Phoenix’s built-in testing framework, to ensure that all components are functioning as expected. Write tests to cover scenarios such as message broadcasting, user joining/leaving the chat room, and error handling.
Conclusion
In this tutorial, we’ve explored the process of building a real-time chat application using Elixir and WebSockets. By harnessing the power of Elixir’s concurrency model and Phoenix’s WebSocket abstraction, we’ve created a highly scalable and responsive chat platform. With further customization and enhancements, you can adapt this application to suit various real-time communication needs in your projects.
Now that you have the foundational knowledge, why not take it a step further and explore advanced features such as user authentication, message persistence, and scalability optimization? The possibilities are endless when you combine the elegance of Elixir with the real-time capabilities of WebSockets.
Happy coding!
External Resources:
- Official Elixir Installation Guide – https://elixir-lang.org/install.html
- Phoenix Framework Documentation – https://hexdocs.pm/phoenix/overview.html
- Example Frontend Code on GitHub – https://github.com/example/real-time-chat-app
Table of Contents
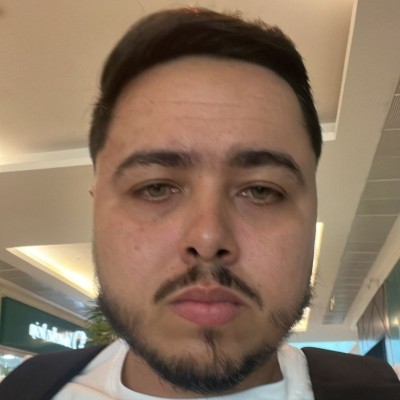
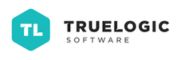