Transforming Code Complexity: Simplify Your Elixir Programming with Macros
As the popularity of Elixir, a dynamic, functional language designed for building scalable and maintainable applications, continues to surge, more companies are looking to hire Elixir developers to harness its power. One of the language’s most powerful, yet misunderstood, features is the macro system. This blog post is all about demystifying Elixir macros and how, by hiring experienced Elixir developers, you can leverage this feature to write powerful code generators.
1. What are Macros in Elixir?
Macros in Elixir are a way of writing code that writes other code, a technique known as metaprogramming. Elixir macros allow us to generate code at compile time, offering possibilities that are hard or impossible to achieve in many other languages.
Let’s put it in simpler terms: Imagine you’re writing a novel. Instead of penning down each word yourself, you decide to hire a ghostwriter who writes chapters based on your instructions. This parallels the scenario when you hire Elixir developers in the world of programming. In this scenario, you’re the product manager, the novel is your program, and the ghostwriter is akin to the Elixir macro—writing parts of your “novel” based on your instructions. Just as you’d hire a talented ghostwriter, it’s crucial to hire skilled Elixir developers to effectively utilize macros for powerful code generation.
2. Why Use Macros?
One might argue that writing straightforward code is better than using macros, which can be harder to understand and debug. That’s a valid point—macros should not be the go-to solution for every problem. However, they become indispensable when you need to eliminate boilerplate code or when you’re building a library for other developers to use. In these cases, macros can help keep code DRY (Don’t Repeat Yourself) and easier to maintain.
3. Macro Basics: Quote and Unquote
Before diving into examples, let’s first familiarize ourselves with two key building blocks of macros in Elixir: `quote` and `unquote`.
3.1 Quote
`quote` gives us the internal representation of Elixir code, which is a form of an Abstract Syntax Tree (AST). Here’s an example:
```elixir IO.inspect(quote(do: 1 + 2)) ```
This will output: `{:+, [context: Elixir, import: Kernel], [1, 2]}`. This output is the AST representation of `1 + 2`.
3.2 Unquote
`unquote` allows us to inject code into a `quote`. Let’s see an example:
```elixir num = 2 IO.inspect(quote(do: 1 + unquote(num))) ```
This will output the same AST as before, as `unquote(num)` allows us to inject the value of `num` into the `quote`.
4. Writing Our First Macro
Let’s start by creating a simple macro that greets a user:
```elixir defmodule Greet do defmacro hello(name) do quote do IO.puts("Hello, " <> unquote(name)) end end end ```
In the `hello` macro, we use `quote` to generate a piece of code that prints a greeting. We then use `unquote(name)` to inject the name parameter into the quoted code. To use this macro, we need to require the module it’s in and then call it:
```elixir defmodule Main do require Greet def greet do Greet.hello("Alice") end end ```
Running `Main.greet()` will print `Hello, Alice`.
5. Example: A Logging Macro
Let’s write a macro that helps eliminate repetitive logging code. Suppose we have several functions where we log the start and end of the function. Without a macro, it might look something like this:
```elixir defmodule MyModule do def my_function(arg1, arg2) do Logger.info("my_function/2 started") # function body Logger.info("my_function/2 ended") end end ```
Now, let’s write a `log_execution` macro that wraps a function with logging statements:
```elixir defmodule Logging do defmacro log_execution(function_ast) do quote do Logger.info(unquote(function_ast) <> " started") unquote(function_ast) Logger.info(unquote(function_ast) <> " ended") end end end ```
Now, we can use `log_execution` to automatically log the start and end of a function:
```elixir defmodule MyModule do require Logging def my_function(arg1, arg2) do Logging.log_execution do # function body end end end ```
The `log_execution` macro helps us keep our code DRY and improves readability.
6. Example: Generating Functions with Macros
Macros can also generate multiple similar functions. Let’s say we need functions to handle different HTTP methods in a web server:
```elixir defmodule Router do def handle_get(path), do: # implementation def handle_post(path), do: # implementation # and so on... end ```
We can use a macro to generate these functions:
```elixir defmodule Router do for method <- ~w(get post put delete) do defmacro unquote(:"handle_#{method}")(path) do quote do # implementation end end end end ```
The `for` loop generates `handle_get`, `handle_post`, `handle_put`, and `handle_delete` functions with a single macro.
Conclusion
Elixir’s macro system is a powerful tool that allows us to write code that generates other code. Although macros can be a bit challenging to wrap your head around initially, they are incredibly useful in certain situations, especially when building libraries or frameworks, and reducing boilerplate code.
As with any powerful tool, it’s important to use macros judiciously. They can make code harder to understand and debug if used inappropriately, and this is one of the reasons to hire Elixir developers who are experienced and skilled in this area. Macros should not be your first solution to a problem. However, when used correctly, macros can make your Elixir code more concise, maintainable, and powerful.
Remember: the key to mastering macros, like anything else in programming, is practice. Hiring seasoned Elixir developers can bring this expertise to your team. So, keep exploring, experimenting, and learning! Happy coding!
Table of Contents
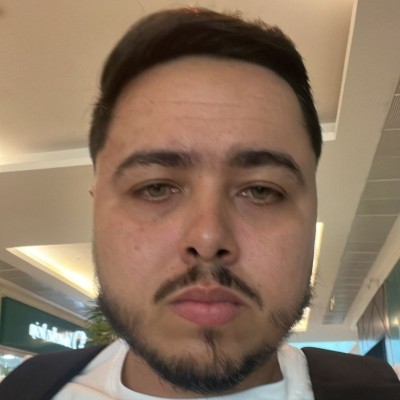
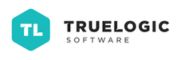