Exploring Elixir’s Concurrency Primitives: Tasks and Agents
In the world of concurrent programming, Elixir stands out as a language designed from the ground up to embrace concurrency and scalability. One of the key features that enable this is its rich set of concurrency primitives, namely Tasks and Agents. In this article, we’ll delve into these powerful tools, exploring their usage, characteristics, and real-world examples.
Understanding Elixir’s Concurrency Primitives
1. Tasks:
Tasks in Elixir are lightweight units of computation that run concurrently with other parts of the program. They are ideal for performing asynchronous operations such as fetching data from external APIs, processing large datasets, or handling background tasks.
```elixir # Example 1: Creating and executing a Task task = Task.async(fn -> do_some_work() end) # Perform other operations concurrently result = Task.await(task) ```
Tasks are highly scalable, as Elixir’s scheduler efficiently manages thousands of them concurrently without sacrificing performance. Additionally, tasks support supervision, fault tolerance, and error handling mechanisms, making them reliable for building fault-tolerant systems.
2. Agents:
Agents provide a simple and convenient way to manage state in concurrent applications. They encapsulate state and expose a set of functions to interact with it concurrently. Agents are often used for managing shared state across multiple processes while ensuring data integrity.
```elixir # Example 2: Creating and updating an Agent {:ok, agent} = Agent.start_link(fn -> initial_state end) Agent.update(agent, fn state -> update_state(state) end) ```
Agents guarantee synchronous updates to their state, ensuring consistency and avoiding race conditions. Moreover, they support error handling and offer mechanisms for monitoring and supervision, enhancing the resilience of concurrent systems.
Real-World Examples
1. Web Scraping with Tasks:
Imagine you’re building a web scraper to extract data from multiple websites concurrently. You can leverage Elixir’s Task module to spawn tasks for each website, fetching data asynchronously and aggregating the results efficiently.
2. State Management with Agents:
Suppose you’re developing a real-time analytics system that tracks user interactions on a website. Agents can be used to manage the state of the analytics dashboard, allowing multiple processes to update and query the data concurrently while ensuring consistency and integrity.
3. Concurrent File Processing:
In scenarios where you need to process large files concurrently, Elixir’s Task module shines. You can spawn tasks to read and process different parts of the file simultaneously, significantly reducing the processing time and improving overall system throughput.
Conclusion
Elixir’s concurrency primitives, Tasks and Agents, empower developers to build highly scalable, fault-tolerant, and performant systems. By leveraging these tools, you can harness the full potential of concurrent programming while ensuring simplicity and reliability in your applications.
To dive deeper into Elixir’s concurrency model and explore advanced topics, check out the official documentation and tutorials:
- [Elixir Task Module Documentation](https://hexdocs.pm/elixir/Task.html)
- [Elixir Agent Module Documentation](https://hexdocs.pm/elixir/Agent.html)
- [Concurrent Programming in Elixir – Official Guide](https://elixir-lang.org/getting-started/mix-otp/concurrency.html)
Start incorporating Tasks and Agents into your Elixir projects today and unlock new possibilities in concurrent programming!
Table of Contents
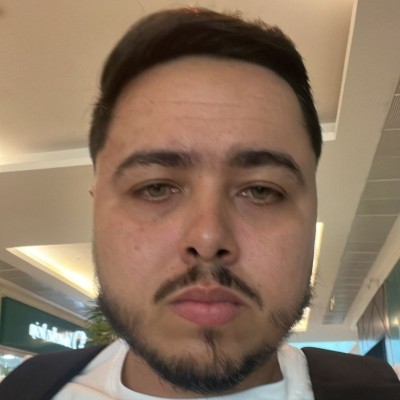
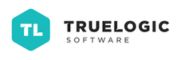