How to create functions in Elixir?
Creating functions in Elixir is a fundamental aspect of Elixir programming, and it’s remarkably straightforward. Functions in Elixir are defined within modules and follow a consistent syntax. Here’s a step-by-step guide on how to create functions in Elixir:
- Module Definition: First, you define a module using the `defmodule` keyword. Modules provide a way to organize your functions and variables. For instance:
```elixir defmodule MathModule do # Functions will be defined here end ```
- Function Definition: Within your module, you can define functions using the `def` keyword followed by the function name and its arguments. For example:
```elixir defmodule MathModule do def add(a, b) do a + b end end ```
In this example, we’ve defined an `add/2` function that takes two arguments, `a` and `b`, and returns their sum.
- Function Body: The function body is enclosed within a `do` block. It contains the logic and operations that the function will perform. The last expression in the block is implicitly returned as the function’s result.
- Pattern Matching: You can use pattern matching in function heads to define multiple clauses of the same function with different argument patterns. Elixir will choose the appropriate clause based on the provided arguments.
```elixir defmodule MathModule do def add(a, b) do a + b end def add(a, b, c) do a + b + c end end ```
In this example, we have two clauses of the `add/2` function, one for two arguments and another for three.
- Calling Functions: To call a function, you simply provide the required arguments within parentheses:
```elixir result = MathModule.add(2, 3) ```
Here, we’re calling the `add/2` function from the `MathModule` module and assigning the result to the `result` variable.
That’s it! With these steps, you can create and use functions in Elixir. Functions are a fundamental building block of Elixir programs, and understanding how to define and use them is essential for effective Elixir development.
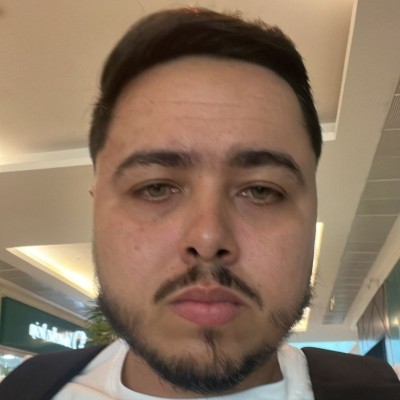
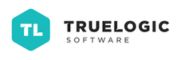