How to create processes in Elixir?
Creating processes in Elixir is a fundamental and powerful aspect of the language, as it allows you to achieve high concurrency and build fault-tolerant applications. In Elixir, processes are lightweight and isolated, and you can create them easily. Here’s how to create processes in Elixir:
- Using the `spawn` Function: The most basic way to create a process in Elixir is by using the `spawn` function. You can pass a function (or an anonymous function) as an argument to `spawn`, and a new process will be spawned to execute that function concurrently. For example:
```elixir pid = spawn(fn -> IO.puts("This is a new process!") end) ```
In this code, a new process is created to execute the anonymous function, and its process identifier (PID) is assigned to the `pid` variable.
- Message Passing: Processes in Elixir communicate by passing messages. You can send messages to a process using the `send` function and receive messages using the `receive` block. This allows processes to exchange data and coordinate their actions. For example:
```elixir send(pid, {:message, "Hello from another process!"}) receive do {:message, msg} -> IO.puts("Received: #{msg}") end ```
Here, we send a message to the `pid` process and then use the `receive` block to handle incoming messages.
- GenServer: Elixir provides the GenServer behavior, a higher-level abstraction for creating server-like processes. GenServer processes maintain state and can handle synchronous and asynchronous requests. To create a GenServer process, you define a module that uses the `GenServer` behavior and implements the required callbacks.
```elixir defmodule MyServer do use GenServer def start_link(_) do GenServer.start_link(__MODULE__, [], name: __MODULE__) end def init(_) do {:ok, []} end end ```
In this example, `MyServer` is a GenServer process that can be started and interacted with using `GenServer.call/3` and `GenServer.cast/2` functions.
Creating processes in Elixir is essential for building concurrent and fault-tolerant applications. By leveraging processes and message passing, you can design systems that effectively handle concurrent tasks, isolate failures, and maintain high availability.
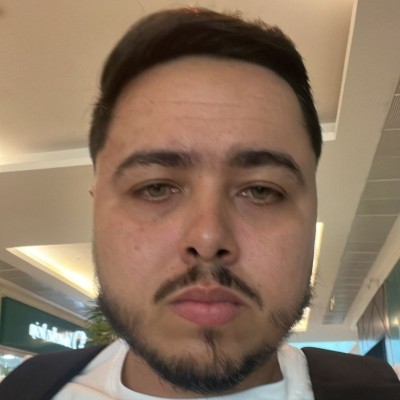
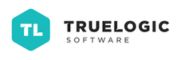