Exploring Elixir’s Enum Module: Powerful Collection Operations
Elixir, the functional programming language built on top of the Erlang VM, is gaining popularity for its elegant syntax, scalability, and fault-tolerance. One of the key features that make Elixir a favorite among developers is its `Enum` module, which provides a plethora of powerful operations for working with collections. In this post, we’ll dive deep into the `Enum` module and explore some of its most useful functions.
Introduction to the Enum Module
The `Enum` module in Elixir is a Swiss Army knife for manipulating collections. Whether you’re working with lists, maps, or other enumerable data structures, the `Enum` module offers a wide range of functions to streamline your code and make complex operations simple.
Map and Reduce: The Workhorses of Functional Programming
At the heart of the `Enum` module are two fundamental functions: `map/2` and `reduce/3`. These functions allow you to transform and aggregate data in a functional, declarative style. Let’s take a closer look at each of them:
1. map/2:
The `map/2` function takes a collection and a function as arguments and applies the function to each element of the collection, returning a new collection with the results. For example:
```elixir list = [1, 2, 3, 4, 5] squared = Enum.map(list, &(&1 * &1)) # Result: [1, 4, 9, 16, 25] ```
2. reduce/3:
The `reduce/3` function, also known as `fold` in other languages, applies a binary function to each element of the collection, accumulating a result along the way. This is incredibly powerful for tasks like summing numbers or finding the maximum value in a collection:
```elixir list = [1, 2, 3, 4, 5] sum = Enum.reduce(list, 0, &(&1 + &2)) # Result: 15 ```
Filtering and Selecting Data
In addition to transforming and aggregating data, the `Enum` module provides functions for filtering and selecting elements from a collection:
1. filter/2:
The `filter/2` function allows you to selectively retain elements from a collection based on a predicate function. For example:
```elixir list = [1, 2, 3, 4, 5] evens = Enum.filter(list, &(&1 % 2 == 0)) # Result: [2, 4] ```
2. take/2:
The `take/2` function returns the first `n` elements from a collection. This is useful for limiting the size of a collection or implementing pagination:
```elixir list = [1, 2, 3, 4, 5] first_three = Enum.take(list, 3) # Result: [1, 2, 3] ```
Conclusion
Elixir’s `Enum` module is a powerful tool for working with collections in a functional, declarative style. By leveraging functions like `map`, `reduce`, `filter`, and `take`, you can write expressive and efficient code that is easy to understand and maintain. Whether you’re processing large datasets or manipulating lists and maps, the `Enum` module has got you covered. Happy coding!
Table of Contents
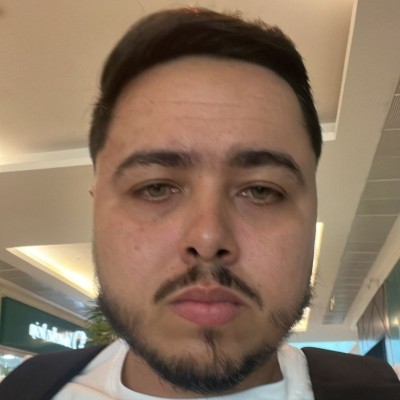
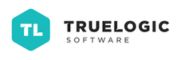