Introduction to Elixir’s Flow: Parallel and Distributed Computing
Elixir is renowned for its ability to handle concurrent and distributed computing gracefully, thanks to its underlying Erlang VM (BEAM). One of Elixir’s standout features for parallel and distributed data processing is the `Flow` module. Flow allows developers to build scalable and efficient data processing pipelines that can handle large datasets and complex computations. This article explores how Elixir’s Flow module can be utilized for parallel and distributed computing, providing practical examples and insights into its powerful capabilities.
Understanding Elixir’s Flow
Flow is a module in Elixir designed to simplify parallel and distributed data processing. It enables developers to process data in parallel streams and distribute the workload across multiple processors or nodes. This results in improved performance and efficiency, particularly when dealing with large volumes of data.
Using Flow for Data Processing
Flow is designed to work seamlessly with enumerables, such as lists and streams, and provides a functional approach to building data processing pipelines. Here are some key aspects and code examples demonstrating how Flow can be employed for parallel and distributed computing.
1. Basic Data Processing with Flow
The simplest use case for Flow is to process data in parallel within a single process. Here’s how you can create a Flow pipeline to process a list of numbers and perform computations in parallel.
Example: Parallel Data Processing
```elixir defmodule ParallelProcessing do use Flow def process_numbers(numbers) do numbers |> Flow.from_enumerable() |> Flow.map(&(&1 * 2)) # Multiply each number by 2 |> Flow.reduce(fn -> 0 end, &(&1 + &2)) # Sum the results |> Enum.to_list() # Collect the results into a list end end numbers = 1..10 result = ParallelProcessing.process_numbers(numbers) IO.inspect(result) # Output will be [110] ```
In this example, `Flow.from_enumerable/1` creates a Flow from a list of numbers. The `Flow.map/2` function processes each number in parallel, and `Flow.reduce/3` aggregates the results.
2. Distributed Data Processing
Flow also supports distributed computing across multiple nodes. This is particularly useful for applications that need to scale horizontally across different machines or cloud environments.
Example: Distributed Data Processing
```elixir defmodule DistributedProcessing do use Flow def process_large_dataset(dataset) do dataset |> Flow.from_enumerable() |> Flow.partition() # Partition the data for distributed processing |> Flow.map(&process_data/1) |> Flow.reduce(fn -> 0 end, &(&1 + &2)) |> Enum.to_list() end defp process_data(data) do # Simulate data processing data * 3 end end dataset = 1..1000 result = DistributedProcessing.process_large_dataset(dataset) IO.inspect(result) # Output will vary based on processing ```
In this example, `Flow.partition/1` partitions the dataset for distributed processing, allowing different nodes to handle different parts of the data concurrently.
3. Handling Backpressure
Flow provides mechanisms to handle backpressure, which is essential when dealing with large volumes of data or varying processing speeds. This ensures that the system remains responsive and avoids overload.
Example: Managing Backpressure
```elixir defmodule BackpressureExample do use Flow def process_stream(stream) do stream |> Flow.from_enumerable() |> Flow.map(&slow_operation/1) |> Flow.buffer(:infinity) # Manage backpressure by buffering |> Enum.to_list() end defp slow_operation(data) do # Simulate a slow operation :timer.sleep(100) data end end stream = 1..50 result = BackpressureExample.process_stream(stream) IO.inspect(result) # Output will vary based on processing ```
Here, `Flow.buffer/2` is used to handle backpressure by buffering the data, preventing the system from being overwhelmed.
4. Integrating Flow with Other Elixir Modules
Flow can be integrated with other Elixir modules and libraries to create complex data processing pipelines. For instance, you can use Flow with GenServer for managing state or with Phoenix channels for real-time data processing.
Example: Integrating with GenServer
```elixir defmodule FlowWithGenServer do use Flow def start_link(_) do GenServer.start_link(__MODULE__, %{}, name: __MODULE__) end def init(state) do {:ok, state} end def handle_info(:process_data, state) do data = [1, 2, 3, 4, 5] processed_data = process_data(data) IO.inspect(processed_data) {:noreply, state} end defp process_data(data) do data |> Flow.from_enumerable() |> Flow.map(&(&1 + 1)) |> Enum.to_list() end end ```
In this example, a GenServer handles data processing with Flow, showcasing how you can integrate Flow with Elixir’s concurrency primitives.
Conclusion
Elixir’s Flow module provides a robust framework for parallel and distributed computing, enabling efficient data processing and improved performance. Whether you’re processing data in parallel within a single machine or distributing tasks across multiple nodes, Flow offers the tools and abstractions needed to handle large datasets and complex computations effectively. By leveraging Flow’s capabilities, you can build scalable and performant data processing pipelines in your Elixir applications.
Further Reading:
Table of Contents
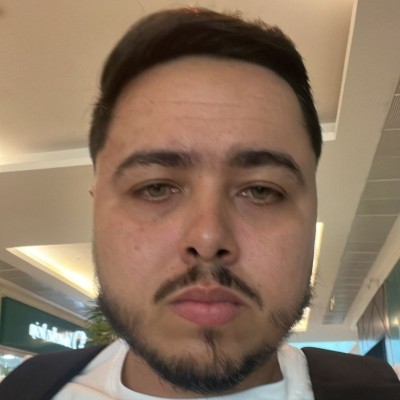
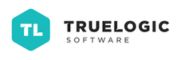