Elixir Q & A
How to handle authentication in a Phoenix application?
Handling authentication in a Phoenix application is a crucial aspect of building secure web applications. Phoenix, being a robust framework, provides several options for implementing authentication. Here’s a high-level overview of the process:
- Select an Authentication Library: Phoenix doesn’t include built-in authentication, so you’ll need to choose an authentication library. Two popular choices are Guardian and Coherence. Guardian is a lightweight and flexible choice, while Coherence provides more features out of the box.
- Install and Configure the Library: Once you’ve chosen a library, add it to your project’s dependencies in the `mix.exs` file and fetch the dependencies using `mix deps.get`. Follow the library’s documentation to configure it for your application. This typically involves setting up encryption keys, session storage, and other options.
- Create a User Model: In most cases, you’ll need a `User` model to represent users in your application. Use Phoenix’s built-in generators or create your user schema with Ecto. Define fields such as email and password_hash for authentication.
- Implement Registration and Login: Create user registration and login functionality. This involves creating routes, controllers, and views to handle user registration, email confirmation (if required), and login. Ensure that passwords are securely hashed before storing them in the database.
- Session Management: Phoenix uses sessions to track user authentication. Implement session management by setting up a session controller to handle user login and logout, and store user-related information in the session.
- Authorization: Beyond authentication, you may also need to implement authorization to control what authenticated users can access. Use role-based access control (RBAC) or other authorization mechanisms to restrict access to certain parts of your application.
- Remember Me and Token-Based Authentication: Some authentication libraries support “remember me” functionality and token-based authentication for APIs. Configure these features as needed, especially if you’re building a web application with persistent login sessions.
- Password Resets: Implement a password reset mechanism that allows users to recover their accounts if they forget their passwords. This typically involves sending reset tokens via email and verifying them.
- Security Considerations: Ensure that your authentication implementation is secure. Protect against common vulnerabilities like Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS). Store sensitive information securely and use HTTPS to encrypt data in transit.
- Testing: Write comprehensive tests for your authentication flows to ensure that they work correctly and securely. Test user registration, login, session management, and authorization.
- Logging and Monitoring: Implement logging to track authentication-related activities and monitor for suspicious behavior. Set up alerts for failed login attempts or unusual activity.
- Documentation: Document your authentication process and policies for internal and external users. Clear documentation helps both developers and end-users understand how authentication works in your application.
By following these steps and leveraging a trusted authentication library, you can implement secure and reliable authentication in your Phoenix application, providing a safe environment for your users to interact with your system.
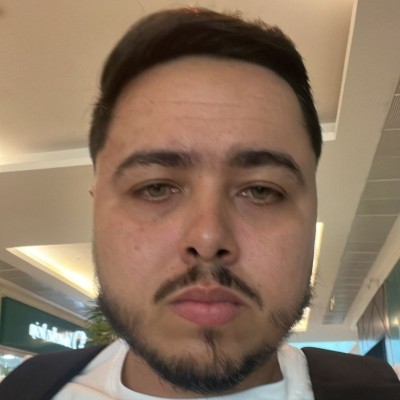
Previously at
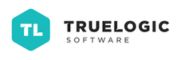
Tech Lead in Elixir with 3 years' experience. Passionate about Elixir/Phoenix and React Native. Full Stack Engineer, Event Organizer, Systems Analyst, Mobile Developer.