Introduction to Elixir’s Mix Umbrella Projects: Managing Multiple Apps
Elixir’s Mix umbrella projects offer a powerful approach to managing multiple applications within a single codebase. This organizational structure is particularly useful for large-scale applications or when developing a suite of interconnected services. By using umbrella projects, you can keep your project modular, organized, and easy to maintain.
Benefits of Mix Umbrella Projects
Umbrella projects in Elixir help in managing complex applications by grouping multiple projects together. This approach provides several benefits:
– Centralized Management: A single repository for all related applications.
– Shared Dependencies: Common libraries and dependencies can be managed in one place.
– Modular Architecture: Allows for better separation of concerns and scalability.
Setting Up a Mix Umbrella Project
Creating an umbrella project is straightforward with Mix, Elixir’s build tool. Here’s a step-by-step guide to setting up your own umbrella project.
1. Creating the Umbrella Project
Start by creating a new umbrella project using Mix.
```bash mix new my_umbrella --umbrella ```
This command sets up a new directory structure with an umbrella application and a `apps` directory where individual applications will reside.
2. Adding Applications to the Umbrella
Within the `apps` directory, you can create new applications. Here’s how to add an application to the umbrella project:
```bash cd my_umbrella mix new app_one mix new app_two ```
This will generate two new applications, `app_one` and `app_two`, each in its respective directory under `apps`.
3. Configuring Dependencies
To manage dependencies across your applications, you can define them in the umbrella project’s `mix.exs` file. This file also allows you to specify applications that should be included in your build.
Example configuration for `mix.exs` in the umbrella root:
```elixir defmodule MyUmbrella.MixProject do use Mix.Project def project do [ apps_path: "apps", deps: deps() ] end def application do [ extra_applications: [:logger] ] end defp deps do [ Define dependencies common to all apps here ] end end ```
4. Building and Running the Umbrella Project
To build and run your umbrella project, use the following commands from the root directory:
```bash mix deps.get mix compile mix phx.server ```
These commands fetch dependencies, compile the project, and start your Phoenix server if you’re using Phoenix for web development.
Managing Applications within the Umbrella
1. Testing Individual Applications
To test an individual application within the umbrella, navigate to the application’s directory and run:
```bash cd apps/app_one mix test ```
2. Adding New Dependencies to an Application
If you need to add dependencies to a specific application, update the `mix.exs` file in that application’s directory.
Example for `apps/app_one/mix.exs`:
```elixir defmodule AppOne.MixProject do use Mix.Project def project do [ app: :app_one, version: "0.1.0", deps: deps() ] end defp deps do [ {:ecto, "~> 3.5"} ] end end ```
3. Sharing Code Between Applications
To share code between applications, you can create a common library within the umbrella project. For instance, create a new application called `common_lib` and use it as a dependency in other applications.
```bash cd apps mix new common_lib ```
Then, add `:common_lib` as a dependency in your other applications’ `mix.exs` files.
Conclusion
Elixir’s Mix umbrella projects provide a robust framework for managing multiple applications within a single codebase. By leveraging this structure, you can achieve better organization, modularity, and efficiency in your development workflow. Whether you’re building a complex application suite or simply want to keep your projects well-organized, umbrella projects can significantly enhance your productivity.
Further Reading
Table of Contents
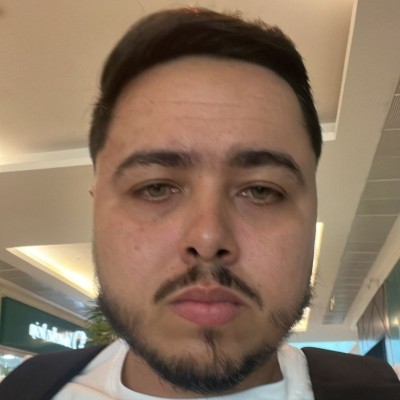
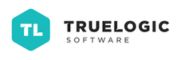