Exploring Elixir’s OTP Behaviors: A Comprehensive Guide
Elixir, a functional programming language built on the Erlang Virtual Machine (BEAM), has gained widespread popularity for its ability to handle concurrent and distributed systems efficiently. One of the cornerstones of Elixir’s concurrent programming capabilities is the OTP (Open Telecom Platform) framework. OTP provides a set of libraries, design principles, and patterns that simplify the development of reliable, fault-tolerant, and scalable applications.
Table of Contents
In this comprehensive guide, we will delve into Elixir’s OTP behaviors – powerful abstractions that encapsulate common patterns for building concurrent systems. We will explore what OTP behaviors are, why they are crucial, and how to effectively use them in your projects.
1. Introduction to OTP Behaviors
1.1. What are OTP Behaviors?
OTP behaviors are predefined sets of callbacks, protocols, and conventions that define common patterns in concurrent programming. These behaviors provide a structured way to create processes with well-defined roles, behaviors, and communication mechanisms. By adhering to these behaviors, developers can create applications that are easier to maintain, understand, and scale.
Elixir provides several built-in OTP behaviors, each tailored for specific use cases. Some of the most widely used behaviors include GenServer, Supervisor, GenStateMachine, and EventManager.
1.2. Why Use OTP Behaviors?
Using OTP behaviors offers numerous advantages, such as:
- Standardization: OTP behaviors establish a common structure for your codebase, making it easier for multiple developers to collaborate on a project.
- Scalability: OTP behaviors encourage the creation of isolated processes that can be distributed across multiple cores or even different nodes, enabling your application to efficiently utilize available resources.
- Fault Tolerance: OTP behaviors help in building systems that can recover from errors and failures gracefully, leading to more robust and reliable applications.
- Code Reusability: By utilizing OTP behaviors, you can create reusable components that adhere to established patterns, reducing duplication and promoting a modular design.
2. OTP GenServer Behavior
2.1. Basics of GenServer
GenServer is one of the fundamental OTP behaviors. It allows you to create processes that maintain state and handle incoming messages concurrently. A GenServer can be used for various purposes, such as managing application state, handling client connections, and more.
Let’s look at a simple example of a counter using the GenServer behavior:
elixir defmodule Counter do use GenServer def start_link(init_count) do GenServer.start_link(__MODULE__, init_count) end def init(init_count) do {:ok, init_count} end def handle_cast(:increment, count) do new_count = count + 1 {:noreply, new_count} end def handle_call(:get_count, _from, count) do {:reply, count, count} end end
In this example, the Counter module uses the GenServer behavior. It initializes the state with a count and defines handlers for both casting an increment message and handling a call to retrieve the count.
2.2. Handling Messages
GenServer processes communicate through messages. You can send messages using cast for asynchronous communication and call for synchronous communication. The process will handle these messages in the appropriate callback functions, namely handle_cast and handle_call.
2.3. State Management
GenServers encapsulate state and ensure that it’s accessible only through defined callback functions. This prevents direct manipulation of the state, promoting a controlled and consistent way of managing data.
2.4. Error Handling
GenServer processes can crash due to various reasons. OTP provides supervision strategies to handle these crashes and restart processes accordingly. This enhances the fault tolerance of your application.
3. OTP Supervisor Behavior
3.1. Supervision Principles
Supervisors are another crucial OTP behavior. They are responsible for starting, stopping, and monitoring child processes. When a supervised process crashes, the supervisor can apply predefined strategies to handle the situation, such as restarting the process.
3.2. Creating a Supervisor
Here’s a simplified example of a supervisor that manages a group of worker processes:
elixir defmodule MySupervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, [], name: __MODULE__) end def init([]) do children = [ worker(WorkerModule, [], restart: :permanent) ] supervise(children, strategy: :one_for_one) end end
In this example, the MySupervisor module uses the Supervisor behavior to manage a worker process defined in WorkerModule. The supervisor’s strategy is set to :one_for_one, which means if one worker crashes, only that worker will be restarted.
3.3. Restart Strategies
Supervisors offer various restart strategies, such as :one_for_one, :one_for_all, :rest_for_one, and :simple_one_for_one. Each strategy defines how child processes are restarted in relation to each other.
3.4. Monitoring Child Processes
Supervisors continuously monitor their child processes. If a child process terminates unexpectedly, the supervisor can take appropriate actions, such as restarting, stopping, or notifying other parts of the application.
4. OTP GenStateMachine Behavior
4.1. Finite State Machines in Elixir
Finite State Machines (FSMs) provide a formal way to model the behavior of systems with a finite number of states and transitions between them. Elixir’s OTP GenStateMachine behavior simplifies the implementation of FSMs.
4.2. Using GenStateMachine
Let’s consider an example of a simple light switch FSM:
elixir defmodule LightSwitch do use GenStateMachine defstates initial: :off, on: :on, off: :off deftransition toggle: %{on: :off, off: :on} deftransition timeout: %{on: :off} end
In this example, the LightSwitch module defines states (:on and :off) and transitions (toggling the light and a timeout transition) using the GenStateMachine behavior.
4.3. Transitions and Actions
Transitions in GenStateMachine are associated with actions. These actions are functions that are called when a transition occurs. This allows you to define the logic associated with state changes.
5. OTP EventManager Behavior
5.1. Building Event-Driven Systems
Event-driven architectures are widely used in applications that need to handle asynchronous events efficiently. Elixir’s OTP provides the EventManager behavior to simplify building such systems.
5.2. Publisher-Subscriber Pattern
The EventManager behavior follows the publisher-subscriber pattern. Components can subscribe to events of interest, and when a publisher emits an event, all subscribed components are notified.
5.3. EventManager in Action
Here’s an example of how to use the EventManager behavior:
elixir defmodule EventExample do use GenServer def start_link do GenServer.start_link(__MODULE__, [], name: __MODULE__) end def init([]) do event_manager = EventManager.start_link(name: EventManager) {:ok, %{event_manager: event_manager}} end def emit_event(event) do EventManager.publish(event, "Event published") end end
In this example, the EventExample module uses the GenServer behavior to create an event manager and emit events.
6. Case Study: Building a Distributed Application
6.1. Combining OTP Behaviors
In real-world applications, combining multiple OTP behaviors can lead to powerful and flexible systems. For instance, you can create a distributed application using supervisors, GenServers, and event managers to ensure fault tolerance, state management, and efficient event handling across nodes.
6.2. Fault Tolerance in Distributed Systems
Distributed systems are prone to network failures and other challenges. By utilizing OTP behaviors, you can design your application to handle these issues gracefully, ensuring uninterrupted operation even in the face of failures.
6.3. Ensuring Consistency
Consistency is crucial in distributed systems. OTP behaviors offer tools for managing data consistency across nodes, allowing you to maintain a coherent state across your application.
7. Best Practices for Using OTP Behaviors
7.1. Choosing the Right Behavior
Selecting the appropriate OTP behavior for a given use case is essential. Carefully evaluate the requirements of your application to determine whether GenServer, Supervisor, GenStateMachine, or EventManager is the best fit.
7.2. Designing for Scalability
When designing with OTP behaviors, keep scalability in mind. Distribute processes across nodes when needed, utilize supervisors to manage worker processes, and design for horizontal scalability by dividing responsibilities among processes.
7.3. Testing and Debugging
Writing tests for your OTP behaviors is crucial to ensure their correctness. Elixir’s built-in testing framework makes it easy to write unit and integration tests for your processes. Additionally, leverage debugging tools and practices to diagnose and address issues effectively.
Conclusion
Elixir’s OTP behaviors empower developers to create robust, fault-tolerant, and scalable concurrent applications. By understanding and effectively using behaviors like GenServer, Supervisor, GenStateMachine, and EventManager, you can build systems that excel in performance, maintainability, and resilience. Whether you’re building a small application or a complex distributed system, the principles and patterns provided by OTP behaviors will undoubtedly be valuable tools in your Elixir programming arsenal. Start exploring OTP behaviors today and unlock the full potential of concurrent programming in Elixir!
Table of Contents
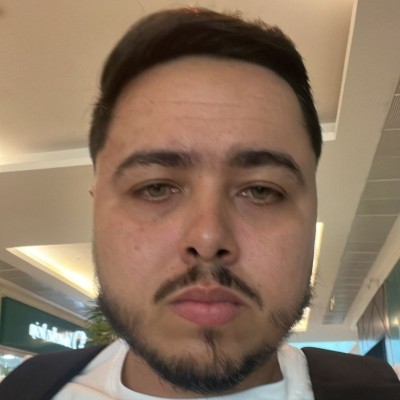
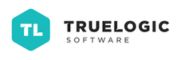