Introduction to Elixir’s Process Registry: Global Process Names
In the vast landscape of programming languages, Elixir stands out as a robust and scalable option, particularly for building distributed and fault-tolerant systems. One of its powerful features is the Process Registry, which allows developers to assign global names to processes, simplifying communication and supervision within an application. In this article, we’ll delve into the intricacies of Elixir’s Process Registry and explore how global process names streamline development.
Understanding Elixir’s Process Registry
In Elixir, processes are the building blocks of concurrent and distributed systems. They operate independently and communicate through message passing. While processes can be identified by their PIDs (Process Identifiers), managing them solely through PIDs can become cumbersome, especially in large-scale applications.
The Process Registry provides a solution by allowing developers to register processes with unique global names. These names can be atoms, making them easy to reference throughout the application. By associating meaningful names with processes, developers can improve code readability and maintainability.
Benefits of Global Process Names
1. Simplified Communication:
With global process names, developers can send messages to processes using their registered names instead of PIDs. This abstraction simplifies message passing and reduces the risk of errors due to PID mismatches.
2. Dynamic Supervision:
Elixir’s supervision trees are fundamental for building fault-tolerant systems. By registering processes with global names, supervisors can dynamically monitor and restart them as needed, enhancing system resilience.
3. Scalability:
In distributed systems, managing processes across multiple nodes can be challenging. Global process names provide a unified interface for interacting with processes regardless of their location, facilitating scalability and fault tolerance.
Examples of Using Global Process Names
Example 1: Worker Processes
```elixir defmodule Worker do use GenServer def start_link(name) do GenServer.start_link(__MODULE__, name, name: name) end def init(name) do {:ok, name} end end # Start worker processes with global names Worker.start_link(:worker1) Worker.start_link(:worker2) # Retrieve worker process by name worker1_pid = Process.whereis(:worker1) ```
Example 2: Supervision
```elixir defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, :ok) end def init(:ok) do children = [ {Worker, [:worker1]}, {Worker, [:worker2]} ] supervise(children, strategy: :one_for_one) end end # Start supervision tree with worker processes MyApp.Supervisor.start_link() ```
Example 3: Dynamic Process Registration
```elixir defmodule RegistryExample do def start_link(name) do Registry.start_link(keys: :unique, name: __MODULE__, owner: Process.whereis(:registry)) Registry.register(__MODULE__, name) end end # Register processes dynamically RegistryExample.start_link(:process1) RegistryExample.start_link(:process2) ```
Conclusion
Elixir’s Process Registry with global process names empowers developers to build scalable and fault-tolerant applications with ease. By abstracting away the complexities of managing processes, developers can focus on designing robust systems that meet the demands of modern distributed computing.
To dive deeper into Elixir’s Process Registry, check out the official documentation [here](https://hexdocs.pm/elixir/Registry.html). For real-world examples and best practices, explore the codebases of popular Elixir libraries like [Phoenix](https://hexdocs.pm/phoenix/), which leverage the Process Registry for building web applications.
Incorporating global process names into your Elixir projects can unlock new possibilities for concurrency, fault tolerance, and scalability. Embrace this powerful feature and elevate your Elixir development experience to new heights. Happy coding!
Table of Contents
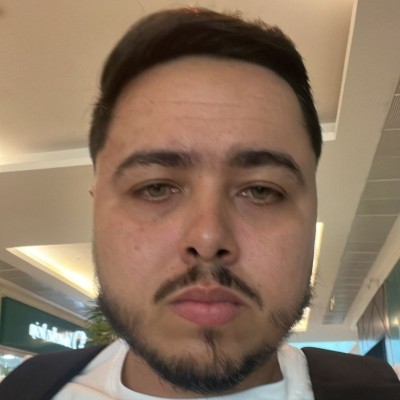
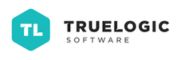